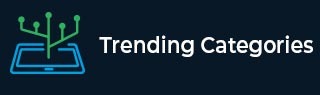
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count elements such that there are exactly X elements with values greater than or equal to X in C++
We are given with an array of integers. The goal is to find the count of elements in the array that satisfy the following condition −
For each element the count of numbers greater than or equal to it present in the array should be exactly equal to it. Excluding the element itself. If element is X then array has exactly X numbers which are greater or equal to X. (Excluding the element).
Input
Arr[]= { 0,1,2,3,4,9,8 }
Output
Elements exactly greater than equal to itself : 1
Explanation − Elements and numbers >= to it −
Arr[0]: 6 elements are >= 0 , 6!=0 count=0 Arr[1]: 5 elements are >= 1 , 5!=1 count=0 Arr[2]: 4 elements are >= 2 , 4!=2 count=0 Arr[3]: 3 elements are >= 3 , 3==3 count=1 Arr[4]: 2 elements are >= 4 , 2!=4 count=1 Arr[4]: 0 elements are >= 9 , 0!=9 count=1 Arr[6]: 1 element is >= 8 , 1!=8 count=1
3 is the only element such that exactly 3 elements are >= to it (4,8,9)
Input
Arr[]= { 1,1,1,1,1 }
Output
Elements exactly greater than equal to itself : 0
Explanation − All elements are equal and count !=1
Approach used in the below program is as follows
The integer array Arr[] is used to store the integers.
Integer ‘n’ stores the length of the array.
Function findcount(int arr[],int n) takes an array and its size as input and returns the count of numbers like X explained earlier.
Variable count is used to store the count of numbers like X.
Initialize ans=0, which will count such numbers.
Traverse the array starting from the first element( index=0 ) using for loop.
Inside for loop traverse from the starting element again, if any arr[j]>=arr[j] such that i!=j, increment the count.
After the end of j loop, compare count with arr[i]. If count==arr[i] (exactly arr[i] elements are >=arr[i], increment the answer ‘ans’
After the end of both for loops return the result present in ‘ans’.
Example
#include <iostream> #include <algorithm> using namespace std; int findcount(int arr[],int n){ sort(arr,arr+n); int count=0; int ans=0; for(int i=0;i<n;i++){ count=0; for(int j=0;j<n;j++){ if(arr[j]>=arr[i] && i!=j) count++; } if(count==arr[i]) ans++; } return ans; } int main(){ int Arr[]= { 0,1,2,3,4,5,6 }; int k=7; int n=sizeof(Arr)/sizeof(Arr[0]); std::cout<<"Elements exactly greater than equal to itself : "<<findcount(Arr,n); return 0; }
Output
Elements exactly greater than equal to itself : 1