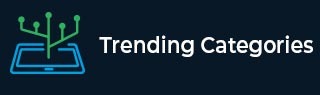
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count elements smaller than or equal to x in a sorted matrix in C++
We are given a matrix of size n x n, an integer variable x, and also, the elements in a matrix are placed in sorted order and the task is to calculate the count of those elements that are equal to or less than x.
Input −
matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {6, 7, 8}} and X = 4
Output −
count is 4
Explanation − we have to match our matrix data with the value x, so the elements less than or equals to x i.e. 4 are 1, 2, 3, 4. So the count is 4.
Input −
matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {6, 7, 8}} and X = 0
Output −
count is 0
Explanation − we have to match our matrix data with the value x, so there is no element that is less than or equals to x. So the count is 0.
Approach used in the below program is as follows
Input the size of the matrix and then create the matrix of size nxn
Start the loop, I from 0 to row size
Inside the loop, I, start another loop j from 0 to column size
Now, check if matrix[i][j] = x, IF yes then increase the count by 1 Else ignore the condition
Return the total count
Print the result.
Example
#include <bits/stdc++.h> using namespace std; #define size 3 //function to count the total elements int count(int matrix[size][size], int x){ int count=0; //traversing the matrix row-wise for(int i = 0 ;i<size; i++){ for (int j = 0; j<size ; j++){ //check if value of matrix is less than or //equals to the x if(matrix[i][j]<= x){ count++; } } } return count; } int main(){ int matrix[size][size] ={ {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; int x = 5; cout<<"Count of elements smaller than or equal to x in a sorted matrix is: "<<count(matrix,x); return 0; }
Output
If we run the above code we will get the following output −
Count of elements smaller than or equal to x in a sorted matrix is: 5