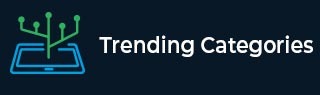
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count characters with same neighbors in C++
We are given a string with, let’s say, str and the task is to compute the count of characters in a string str having the same neighbors and that will include both left and right side of a character in a string. Also, in this scenario the first and last character in a string will always be considered as they have only one adjacent character.
For Example
Input − string str = “poiot” Output − count is 3
Explanation − In the given string characters p, t and i are having the same neighbors so the count will be increased to 3.
Input − string str = “nitihig” Output − count is 4
Explanation − In the given string characters n, t, h and g are having the same neighbors so the count will be increased to 4.
Approach used in the below program is as follows
Input the string in a variable let’s say str
Calculate the length of string str using length() function that will return an integer value as per the number of letters in the string including the spaces.
If the length of the string is less than or equals 2 then return the count as the length of the string because they all will be counted.
If the length of the string is greater than 2, initialise the count with 2.
Now, start the loop with i to 1 till i is less than (length-1)
Inside the loop, check if (str[i-1] = str[i+1]) then increase the count by 1
Now return the total value of count
Print the result.
Example
#include <iostream> using namespace std; // To count the characters int countChar(string st){ int size = st.length(); if (size <= 2){ return size; } int result = 2; // Traverse the string for (int i = 1; i < size - 1; i++){ // Increment the count by 1 if the previous // and next character is same if (st[i - 1] == st[i + 1]){ result++; } } // Return result return result; } int main(){ string st = "poiot"; cout <<"count is " <<countChar(st); return 0; }
Output
If we run the above code it will generate the following output
count is 3