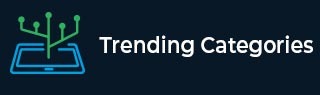
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Converting alphabets to Greek letters in JavaScript
Problem
We are required to write a JavaScript function that takes in a string of uppercase English alphabets, str, as the first and the only argument.
Consider the following mapping between English and Greek letters −
A=α (Alpha) B=β (Beta) D=δ (Delta) E=ε (Epsilon) I=ι (Iota) K=κ (Kappa) N=η (Eta) O=θ (Theta) P=ρ (Rho) R=π (Pi) T=τ (Tau) U=μ (Mu) V=υ (Upsilon) W=ω (Omega) X=χ (Chi) Y=γ (Gamma)
For all the alphabets that have a Greek mapping our function should create a new string in which the English letter is replaced by corresponding Greek letter and if there exists no mapping we should persist with the English alphabet.
For example, if the input to the function is −
Input
const str = 'PLAYING';
Output
const output = 'ρlαγιηg';
Example
Following is the code −
const str = 'PLAYING'; const convertLang = (str) => { const map = { a:'α',b:'β',d:'δ',e:'ε', i:'ι',k:'κ',n:'η',o:'θ', p:'ρ',r:'π',t:'τ',u:'μ', char:'υ',w:'ω',x:'χ',y:'γ' }; return str.replace(/./g, char => { if (map[char.toLowerCase()]){ return char === char.toUpperCase() ? map[char.toLowerCase()] : map[char]; }; return char.toLowerCase(); }); }; console.log(convertLang(str))
Output
Ρlαγιηg
Advertisements