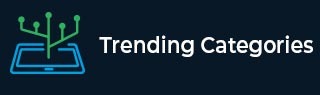
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert String to IntStream in Java
If you have a string and you want to convert it into IntStream with ASCII values, then it can be easily achieved using the below code.
To work with IntStream class, you need to import the following package:
import java.util.stream.IntStream;
Let’s say the following is our string:
String str = "Benz";
Convert the above string to IntStream:
IntStream stream = str.chars();
The following is an example to convert String to IntStream in Java:
Example
import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { String str = "Benz"; System.out.println("String to be converted = " + str); System.out.println("String converted to IntStream (ASCII Values) = "); IntStream stream = str.chars(); stream.forEach(System.out::println); } }
output
String to be converted = Benz String converted to IntStream (ASCII Values) = 66 101 110 122
Advertisements