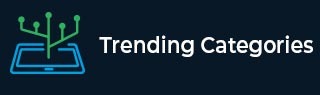
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert a given Binary Tree to Doubly Linked List (Set 1) in C++
In this tutorial, we will be discussing a program to convert a binary tree to a doubly linked list.
For this we will be provided with a binary tree. Our task is to convert it into a doubly linked list such that the left and right pointers become the previous and next pointers. Also the sequential order of the doubly linked list must be equal to the inorder traversal of the binary tree.
For this we are having a very straight forward approach. We will be traversing the binary tree in in order way making the nodes of the doubly linked list along with and finally making the left and right to be the previous and next nodes respectively.
Example
#include <iostream> using namespace std; //node structure of binary tree struct node{ int data; node* left; node* right; }; //traversing and making nodes for //doubly linked list void binarytodll(node *root, node **head){ if (root == NULL) return; static node* prev = NULL; //converting left subtree binarytodll(root->left, head); if (prev == NULL) *head = root; else { root->left = prev; prev->right = root; } prev = root; //converting right subtree binarytodll(root->right, head); } //allocating a new node node* newNode(int data) { node* new_node = new node; new_node->data = data; new_node->left = new_node->right = NULL; return (new_node); } //printing nodes of doubly linked list void print_dll(node *node){ while (node!=NULL) { cout << node->data << " "; node = node->right; } } int main(){ node *root = newNode(10); root->left = newNode(12); root->right = newNode(15); root->left->left = newNode(25); root->left->right = newNode(30); root->right->left = newNode(36); node *head = NULL; binarytodll(root, &head); print_dll(head); return 0; }
Output
25 12 30 10 36 15
Advertisements