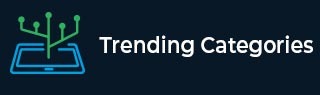
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert a Binary Tree to a Circular Doubly Link List in C++
In this tutorial, we will be discussing a program to convert a binary tree to a circular doubly linked list.
For this, we will be provided with a binary tree. Our task will be to convert the left and right nodes to the left and right elements respectively. And take the inorder of the binary tree to be the sequence order of the circular linked list
Example
#include<iostream> using namespace std; //node structure of the binary tree struct Node{ struct Node *left, *right; int data; }; //appending rightlist to the end of leftlist Node *concatenate(Node *leftList, Node *rightList){ //if one list is empty return the other list if (leftList == NULL) return rightList; if (rightList == NULL) return leftList; Node *leftLast = leftList->left; Node *rightLast = rightList->left; //connecting leftlist to rightlist leftLast->right = rightList; rightList->left = leftLast; leftList->left = rightLast; rightLast->right = leftList; return leftList; } //converting to circular linked list and returning the head Node *bTreeToCList(Node *root){ if (root == NULL) return NULL; Node *left = bTreeToCList(root->left); Node *right = bTreeToCList(root->right); root->left = root->right = root; return concatenate(concatenate(left, root), right); } //displaying circular linked list void print_Clist(Node *head){ cout << "Circular Linked List is :\n"; Node *itr = head; do{ cout << itr->data <<" "; itr = itr->right; } while (head!=itr); cout << "\n"; } //creating new node and returning address Node *newNode(int data){ Node *temp = new Node(); temp->data = data; temp->left = temp->right = NULL; return temp; } int main(){ Node *root = newNode(10); root->left = newNode(12); root->right = newNode(15); root->left->left = newNode(25); root->left->right = newNode(30); root->right->left = newNode(36); Node *head = bTreeToCList(root); print_Clist(head); return 0; }
Output
Circular Linked List is : 25 12 30 10 36 15
Advertisements