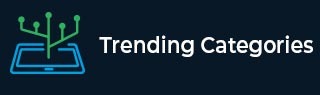
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Complete Guide on How to build Video Player in Android
What is a Video Player in Android?
In this tutorial, we are going to learn on How to build a video player in our android application using Kotlin as a programming language.
Video player is a widget present in android which is used to access video files within android application. With the help of this video player we can view the video within our android application.
Implementation of Video Player
We will be creating a simple application in which we will be using a video view to play video within our android application. We will be following a step by step guide to implement a toast message in an android application.
Step 1 : Creating a new Project in Android Studio
Navigate to Android studio as shown in below screen. In the below screen click on New Project to create a new Android Studio Project.
After clicking on New Project you will get to see the below screen.
Inside this screen we have to simply select Empty Activity and click on Next. After clicking on next you will get to see the screen below.
Inside this screen we have to simply specify the project name. Then the package name will be generated automatically.
Note − Make sure to select the Language as Kotlin.
After specifying all the details click on Finish to create a new Android studio project.
Once our project has been created we will get to see 2 files which are open i.e activity_main.xml and MainActivity.kt file.
Step 2 : Working with activity_main.xml
Navigate to activity_main.xml. If this file is not visible. To open this file. In the left pane navigate to app>res>layout>activity_main.xml to open this file. After opening this file. Add the below code to it. Comments are added in the code to get to know in detail.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <!-- creating a video view on below line--> <VideoView android:id="@+id/idVideoView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" /> </RelativeLayout>
Explanation − In the above code the root element is a Relative layout in android. This layout is a view group which is used to align all the elements within it relative to each other. We can relatively align all elements within Relative Layout with the help of ids or positions.
Inside this relative layout we are creating our video view which is used to play videos within our android application. Inside this video view firstly we are specifying the unique id so that we can use that to load our video. Then we are specifying height and width for our video view. Then we are aligning our video view to the center of the screen.
At last we are adding a closing tag for our Relative Layout as the text view and button is enclosed in our relative layout.
Step 3 : Adding your video in Android Studio Project
For adding your video inside the android application we have to simply copy the video which we want to play inside our video view. Then we have to navigate to our android studio project. Inside our project navigate to app>res>Right click on it>New>Directory and specify the name of it as raw. After that simply right click on that directory and click on paste option to add your video inside our project. Make sure to give the video name as “video” because we will be using the same name while using this video inside our project.
Step 4 : Working with MainActivity.kt
Navigate to MainActivity.kt. If this file is not visible. To open this file. In the left pane navigate to app>java>your app’s package name>MainActivity.kt to open this file. After opening this file. Add the below code to it. Comments are added in the code to get to know in detail.
package com.example.gptapp import android.net.Uri import android.os.Bundle import android.widget.MediaController import android.widget.VideoView import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { // creating variables for video view on below line. lateinit var videoView: VideoView override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // initializing variable for video view on below line. videoView = findViewById(R.id.idVideoView) // creating and initializing variable for media controller on below line. val mediaController = MediaController(this) // setting media controller anchor view to video view on below line. mediaController.setAnchorView(videoView) // on below line we are getting uri for the video file which we are adding in our project. val uri: Uri = Uri.parse("android.resource://" + packageName + "/" + R.raw.video) //on below line we are setting media controller for our video view. videoView.setMediaController(mediaController); // on below line we are setting video uri for our video view. videoView.setVideoURI(uri); // on below line we are requesting focus for our video view. videoView.requestFocus(); // on below line we are calling start method to start our video view. videoView.start(); } }
Explanation − In the above code for the MainActivity.kt file. Firstly we are creating a variable for the video view.
Now we will get to see the onCreate method. This is the default method of every android application. This method is called when the application view is created. Inside this method we are setting the content view i.e the layout file named activity_main.xml to set the UI from that file.
After specifying the view we are initializing our Video View variable named as videoView with its unique id which we have given in the activity_main.xml file.
After initializing our videoView we are creating and initializing variables for the media controller. Media Controller is used to control the media playback. It is used to play and pause the video. We can move video forward and backward using the media controller.After initializing our media controller, we are setting the anchor view for the media controller as video View. Then we are creating a uri variable where we will be generating the path for the video file which we have added inside our project's raw folder. After initializing our video uri we are setting the media controller for our video view to control the video being played in our video view. Then we are loading video uri inside our video view. At last we are requesting focus for our video view and then starting the video view by calling start method.
After adding the above code now we have to simply click on the green icon in the top bar to run our application on a mobile device.
Note − Make sure you are connected to your real device or emulator.
Conclusion
In the above tutorial we learn What is a video player in android and How we can use it inside our android application to play videos within our android application.