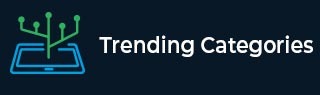
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Coin Path in C++
Suppose we have an array A (index starts at 1) with N numbers: A1, A2, ..., AN and another integer B. The integer B denotes that from any index is i in the array A, we can jump to any one of the places in the array A indexed i+1, i+2, …, i+B if this place can be jumped to. Also, if we step on the index i, we have to pay Ai amount of coins. If Ai is -1, it means we can’t jump to the place indexed i in the array.
Now, when we start from the place indexed 1 in the array A, and our aim is to reach the place indexed N using the minimum coins. We have to return the path of indexes (starting from 1 to N) in the array we should take to get to the place indexed N using minimum coins. If we have multiple paths with the same cost, we have to find the lexicographically smallest such path. And if we have no such possible path to reach the place indexed N then we will return an empty array.
So, if the input is like [1,2,4,-1,2], 2, then the output will be [1,3,5]
To solve this, we will follow these steps −
n := size of A
Define an array ret
Define an array cost of size n, and fill this with inf
Define an array next of size n, and fill this with -1
if not n is non-zero or A[n - 1] is same as -1, then −
endPoint := n - 1
cost[n - 1] = A[n - 1]
for initialize i := n - 2, when i >= 0, update (decrease i by 1), do −
if A[i] is same as -1, then −
Ignore following part, skip to the next iteration
for j in range i + 1 to minimum of (n - 1) and i + B, increase j by 1 −
if cost[j] + A[i] < cost[i], then −
cost[i] := cost[j] + A[i]
next[i] := j
endPoint := i
if endPoint is not equal to 0, then −
return empty array
for endPoint is not equal to -1, update endPoint = next[endPoint], do −
insert endPoint + 1 at the end of ret
return ret
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; void print_vector(vector<auto> v){ cout << "["; for(int i = 0; i<v.size(); i++){ cout << v[i] << ", "; } cout << "]"<<endl; } class Solution { public: vector<int> cheapestJump(vector<int>& A, int B) { int n = A.size(); vector <int> ret; vector <int> cost(n, 1e9); vector <int> next(n, -1); if(!n || A[n - 1] == -1) return {}; int endPoint = n - 1; cost[n - 1] = A[n - 1]; for(int i = n - 2; i >= 0; i--){ if(A[i] == -1) continue; for(int j = i + 1 ; j <= min(n - 1, i + B); j++){ if(cost[j] + A[i] < cost[i]){ cost[i] = cost[j] + A[i]; next[i] = j; endPoint = i; } } } if(endPoint != 0) return {}; for(;endPoint != - 1; endPoint = next[endPoint]){ ret.push_back(endPoint + 1); } return ret; } }; main(){ Solution ob; vector<int> v = {1,2,4,-1,2}; print_vector(ob.cheapestJump(v, 2)); }
Input
{1,2,4,-1,2}, 2
Output
[1, 3, 5, ]