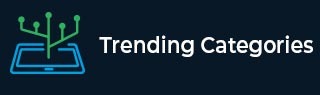
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Class Keyword in JavaScript
JavaScript classes, introduced in ES6, are syntactical sugar over JavaScript prototype-based inheritance. Classes are in fact "special functions". You can define classes in JavaScript using the class keyword using the following syntax −
class Person { // Constructor for this class constructor(name) { this.name = name; } // an instance method on this class displayName() { console.log(this.name) } }
This is essentially equivalent to the following declaration −
let Person = function(name) { this.name = name; } Person.prototype.displayName = function() { console.log(this.name) }
This class can also be written as a class expressions. The above format is a class declaration. The following format is a class expression −
// Unnamed expression let Person = class { // Constructor for this class constructor(name) { this.name = name; } // an instance method on this class displayName() { console.log(this.name) } }
No matter how you define the classes as mentioned above, you can create objects of these classes using the following −
Example
let John = new Person("John"); John.displayName();
Output
John
You can read in depth about JS classes and the class keyword at https://www.tutorialspoint.com/es6/es6_classes.htm.
Advertisements