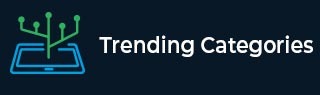
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check whether the vowels in a string are in alphabetical order or not in Python
Suppose we have a string s. We have to check whether the vowels present in s are in alphabetical order or not.
So, if the input is like s = "helloyou", then the output will be True as vowels are e, o, o, u all are in alphabetical order.
To solve this, we will follow these steps −
- character := character whose ASCII is 64
- for i in range 0 to size of s - 1, do
- if s[i] is any one of ('A','E','I','O','U','a','e','i','o','u'), then
- if s[i] < character, then
- return False
- otherwise,
- character := s[i]
- if s[i] < character, then
- if s[i] is any one of ('A','E','I','O','U','a','e','i','o','u'), then
- return True
Let us see the following implementation to get better understanding −
Example Code
def solve(s): character = chr(64) for i in range(len(s)): if s[i] in ['A','E','I','O','U','a','e','i','o','u']: if s[i] < character: return False else: character = s[i] return True s = "helloyou" print(solve(s))
Input
"helloyou"
Output
True
Advertisements