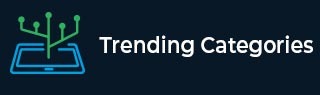
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check whether an array can fit into another array by rearranging the elements in the array
From the problem statement, we can understand that given two arrays, we have to check whether the first array can fit into the second array.
In the real world, there are many instances where we need to check whether an array can fit into another array by rearranging the elements in the array.
For a variety of reasons, programmers may need to reorder the items of an array to see if they can fit into another array. Memory management in computer programming is one such reason. When working with huge amounts of data, it is frequently more effective to use arrays to store that data; but, due to memory constraints, it may be required to arrange arrays in a specific way in order to avoid memory constraints.
Explanation
Let’s try to decode the problem.
Suppose you have two arrays: array A with size n and array B with size m, where m is greater than or equal to n. The task is to check whether it is possible to rearrange the elements of array A in any order such that array A can be completely contained within array B.
In other words, every element of array A must be present in array B, and in the same order as they appear in array A. However, there can be additional elements in array B that are not present in array A.
For example, let's say array A contains the elements [3,2,1] and array B contains the elements [2, 1, 3, 4, 5]. We can rearrange the elements in array A to get [3, 2, 1], which can then be completely contained within array B as follows −
On the other hand, if array A contains the elements [1, 2, 3] and array B contains the elements [2, 3, 4, 5], we cannot rearrange the elements in array A to fit completely within array B, since the element 1 is not present in array B.
Therefore, the function to check whether array A can fit into array B by rearranging the elements would return False in this case.
Approach
Let’s decode the entire program into step by step algorithm.
Sort the two arrays in ascending order.
Compare the elements of the two arrays, starting with the first entry in each array.
Move on to the next element in both arrays if the smaller array's element is less or equal to its equivalent element in the larger array.
Return "false" if the smaller array's element is bigger than its equivalent element in the larger array because the smaller array can't fit inside the larger array.
Return "true" if all of the smaller array's items are less or equal to their corresponding elements in the larger array since the smaller array can fit inside the larger array.
Note − The complexity of this algorithm is O(n log n), where n is the size of the arrays, due to the sorting step.
Example
C++ Code Implementation: Check whether an array can fit into another array by rearranging the elements in the array
#include <iostream> #include <algorithm> #include <vector> using namespace std; bool can_fit(vector<int>& arr_1, vector<int>& arr_2) { //base case if(arr_1.size() > arr_2.size()) return false; // Sort both arrays sort(arr_1.begin(), arr_1.end()); sort(arr_2.begin(), arr_2.end()); // Check if arr_1 can fit into arr_2 int i = 0, j = 0; while (i < arr_1.size() && j < arr_2.size()) { if (arr_1[i] <= arr_2[j]) { i++; j++; } else { return false; } } return true; } int main() { vector<int> A, B; A.push_back(2); A.push_back(5); A.push_back(7); A.push_back(9); A.push_back(10); B.push_back(1); B.push_back(3); B.push_back(5); B.push_back(7); B.push_back(9); B.push_back(9); B.push_back(10); // Check whether B can fit into A if (can_fit(A, B)) { cout << "Array A can fit into array B by rearranging the elements." << endl; } else { cout << "Array A cannot fit into Array B by rearranging the elements." << endl; } return 0; }
Output
Array A cannot fit into array B by rearranging the elements.
Complexities
Time complexity: O(n log n), As in this code first we sort both the arrays and also perform one iteration.
Space complexity: O(n), As we are storing elements of two vectors in memory.
Conclusion
In this article, we have tried to explain the approach to check whether an array can fit into another array. I hope this article helps you to learn the concept in a better way.