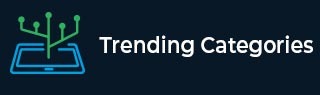
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check whether a given number is Polydivisible or Not
The problem statement includes checking whether a given number is Polydivisible or not for any given integer N. A polydivisible number, also known as magic number, is a number following a unique pattern. The number created by first p digits of the given number should always be divisible by p and there should not be any leading zeros in the given number. If a number satisfies these properties, it is a Polydivisible number, else it is not. Here, p should be in range (1, total digits in the given number). Let’s understand the concept of polydivisible number with an example:
Let's assume a number to be 5432.
Since, it does not begin with a zero it satisfies the first property. Now let’s check for other properties. The number formed by the first two digits of the given number is 54. It is divisible by 2. Similarly the number formed by the first 3 digits of the given number is 543 which is divisible by 3. Further, the number formed by the first 4 digits of the number is divisible by 4. Hence, it is a polydivisible number. Now let's check for number 82325. The number doesn’t start with 0. And the first two digits of the number makes 82 which is divisible by 2. But the first 3 digits of the number makes 823 which is not divisible by 3.
Though, the first 4 digits of the number is divisible by 4 which is 8232 and the first 5 digits of the number is also divisible by 5. Still, it is not a polydivisible number because it does not follow the criteria for a number to be a polydivisible number as the first 3 digits of the number is not divisible by 3. The number formed by the first p digits of the given number should always be divisible by p until p is equal to the number of digits in the given number starting from 2. Our task in the problem includes that we will be given any integer N and we need to check if it is a polydivisible number or not and print accordingly.
For example,
INPUT : N=861
OUTPUT : The number 861 is a polydivisible number.
Explanation : Since every p digit of the number is divisible by p for p>1 and p<=number of digits in the number.
INPUT : N=42587
OUTPUT : The number 42587 is not a polydivisible number.
Explanation : Since the criteria for being a polydivisible number is not followed for p=3. The number formed by the first 3 digits of the number is 425 which is not divisible by 3.
Below is the algorithm that we will use to solve the given problem.
Algorithm
The logic to solve this problem is quite simple. The step-by-step illustration of the algorithm −
We will take out all the digits of the given number N and store them.
We will use an array to store all the digits. We will extract digits using modulo function and divide the number by 10 at every step.
Since the digits stored in the array will be in reverse order so we will reverse the array using reverse() library.
Now we will check for the number if it is a polydivisible number or not using a for loop.
Iterate in a for loop to check for every p digit if it is divisible by p. If it satisfies for every value of p until p is equal to the number of total digits in the given number then, it is a polydivisible number.
Approach
Initialise a function to check whether a number is polydivisible or not.
Initialise an array and store all the digits of the given number N in the array. The array should be of size $\mathrm{\log_{10}{N}\:+\:1}$ as it will always be equal to the number of digits in N. Also typecast the value of $\mathrm{\log_{10}{N}}$ into int to avoid decimal values.
Reverse the array to get the digits in the right order.
Iterate in a for loop from int i=1 to i<size of the array and check if the number formed by the i digits is divisible by i+1 because of zero indexing in the array.
If the number formed by i digits is not divisible by i+1, return false. Else we will return true after all iterations as it satisfies the condition for all the cases.
Print the number is a polydivisible number if function polydivisible returns true and if polydivisible returns false print that the number is not a polydivisible number.
Example
The C++ implementation of the above approach −
#include <bits/stdc++.h> using namespace std; //function to check the conditions of the polydivisible number bool polydivisible(int N){ int d[(int)log(N)+1]={0}; //to store every single digits of the number int x=0; //for storing the index value of array while(N>0){ int a = N%10; //using modulo we can get the last digit of the number d[x]=a; //storing each digit of the number in the array N = N/10; //updating the number by remaining number x++; //increase the index by 1 } int s=sizeof(d)/sizeof(d[0]); reverse(d,d+x); //reversing the array to make right order of digits N=d[0]; for(int i=1;i<x;i++){ //checking the conditions for polydivisible number N = N * 10 + d[i]; //number formed by i digits if(N%(i+1)!=0){ //checking if it is divisible by the number of digits return false; //if not divisible store false in a and break the loop } } return true; } int main(){ int N=522589; if(polydivisible(N)){ //if the function returns true cout<<"The number is a polydivisible number"<<endl; } else { //if the function returns false cout<<"The number is not a polydivisible number"<<endl; } N=9216543; if(polydivisible(N)){ cout<<"The number is a polydivisible number"<<endl; } else { cout<<"The number is not a polydivisible number"<<endl; } return 0; }
Output
The number is not a polydivisible number The number is a polydivisible number
Time Complexity : O($\mathrm{\log_{10}{N}}$)
Space Complexity : O($\mathrm{\log_{10}{N}}$)
Conclusion
We discussed in this article to check whether a given number N is polydivisible or not using an array where we store every digit of the given number in the array and then check for every number formed by p digits of the number if it is divisible by p or not. I hope you find this article helpful in solving your doubts regarding the concept.