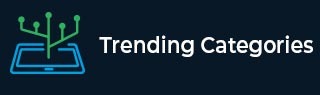
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if product of digits of a number at even and odd places is equal in Python
Suppose we have a number n. We have to check whether the product of odd placed digits and the even placed digits are same or not.
So, if the input is like n = 2364, then the output will be True as product of odd placed numbers are 2 * 6 = 12 and product of even placed numbers are 3 * 4 = 12 which are same.
To solve this, we will follow these steps −
- if num < 10, then
- return False
- odd_place := 1, even_place := 1
- while num > 0, do
- d := last digit of num
- odd_place := odd_place * d
- num := quotient of (num/10)
- if num is same as 0, then
- break
- d := last digit of num
- even_place := even_place * d
- num := quotient of (num/10)
- if odd_place is same as even_place, then
- return True
- return False
Example
Let us see the following implementation to get better understanding −
def solve(num): if num < 10: return False odd_place = 1 even_place = 1 while num > 0: d = num % 10 odd_place *= d num = num//10 if num == 0: break d = num % 10 even_place *= d num = num//10 if odd_place == even_place: return True return False num = 2364 print(solve(num))
Input
2364
Output
True
Advertisements