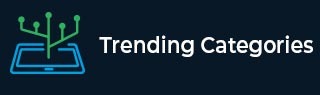
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if items in an array are consecutive but WITHOUT SORTING in JavaScript
In the above statement we are asked to check if items in an array are consecutive without sorting the array with the help of javascript functionalities. We can solve this problem with some basic functionalities of javascript. Let us see how to do this!
What are Consecutive Items in an Array ?
Consecutive elements means every next item should be greater or smaller to the previous element in the sequence. For example we have an array [1, 2, 3, 4, 5], So here we can see that the array contains all the consecutive items as they are in increasing order so the output should be true because they are consecutive.
Example
Input
11 12 13
Output
True
Input
21 11 10
Output
False
Logic for The Above Problem
Before solving the above problem statement, we need to understand the logic behind it to create an algorithm for it.
As we have discussed above that consecutive items should be in increasing order or in decreasing order. So to make sure that the sequence given is these orders, find out the maximum and minimum element of the array. Then we will check the length of the array if the array has only one element then return false otherwise keep forwarding. After that with the help of a for loop we will iterate all the elements and check the condition for each element.
At last we will give the result in the form of boolean. If the sequence follows ascending or descending order then show the result as true otherwise false.
Algorithm
Step 1 − Begin with defining the function called consecutive and pass an input array in it.
Step 2 − Forwarding to the above step, find out the minimum and maximum of the input array.
Step 3 − After that check if the difference of max and min is not equal to the length of the input array. If the condition satisfies then return false.
Step 4 − At the fourth step initialize a for loop and run this loop until the length of the array.
Step 5 − Lastly, show the result as true or false for the given array.
Example
//define a function to check elements are consecutive or not function consecutive(arr) { let min = Math.min(...arr); let max = Math.max(...arr); if (max - min !== arr.length - 1) { return false; } for (let i = 0; i < arr.length; i++) { if (arr.indexOf(min + i) === -1) { return false; } } return true; } //define different arrays with different elements const arr1 = [1, 2, 3, 4, 5]; const arr2 = [13, 12, 11, 10]; const arr3 = [8, 4, 5, 6, 7, 10]; console.log(consecutive(arr1)); console.log(consecutive(arr2)); console.log(consecutive(arr3));
Output
true true false
Algorithm − Without for loop
Step 1 − Begin with finding the minimum and maximum elements in the array.
Step 2 − Forwarding to the first step, calculate the expected sum of consecutive elements.
Step 3 − After the second step calculate the actual sum of elements in the array using Javascript’s reduce method.
Step 4 − At the end compare the sum of step 2 and step 3 to find out if the array contains consecutive items.
Example
//define a function to check elements function consecutive(arr) { const min = Math.min(...arr); const max = Math.max(...arr); const expectedSum = (max - min + 1) * (max + min) / 2; const actualSum = arr.reduce((sum, num) => sum + num, 0); return expectedSum === actualSum; } const arr1 = [13, 12, 11, 10]; const arr2 = [8, 4, 5, 6, 7, 10]; const arr3 = [1, 2, 3, 4, 5]; console.log(consecutive(arr1)); console.log(consecutive(arr2)); console.log(consecutive(arr3));
Output
true false true
Complexity
As we have seen two methods to solve this issue. For the first method which is using a for loop and this loop is running till the length of the array. So the time complexity will be O(n) and space complexity for this code will be O(1) because it is storing a boolean value which takes constant space. For the second method, time complexity is O(n) because the function iterates through all the elements of the array. And both Math.min and Math.max function also takes O(n) time. And space complexity is O(1) because storing a boolean value.
Conclusion
We have learned how to check that the elements in the array are consecutive or not. We created two kinds of algorithms and have seen different outputs for different array inputs. And time and space complexity for both the methods are same O(n) and O(1).