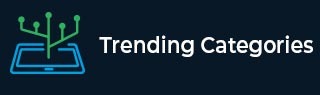
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if it is possible to sort an array with conditional swapping of adjacent allowed in Python
Suppose we have an unordered array of numbers called nums and all elements are in range 0 to n-1. We can swap adjacent elements in nums as many times as required but only when the absolute difference between these element is 1. We have to check whether we can sort the nums or not.
So, if the input is like nums = [1, 0, 3, 2, 5, 4], then the output will be True as we can swap these pairs [(1, 0), (3, 2), (5, 4)] to sort [0, 1, 2, 3, 4, 5].
To solve this, we will follow these steps −
- for i in range 0 to size of nums - 2, do
- if nums[i] > nums[i+1], then
- if nums[i] - nums[i+1] is same as 1, then
- exchange nums[i] and nums[i+1]
- otherwise,
- return False
- if nums[i] - nums[i+1] is same as 1, then
- if nums[i] > nums[i+1], then
- return True
Example
Let us see the following implementation to get better understanding −
def solve(nums): for i in range(len(nums) - 1): if nums[i] > nums[i+1]: if nums[i] - nums[i+1] == 1: nums[i], nums[i+1] = nums[i+1], nums[i] else: return False return True nums = [1, 0, 3, 2, 5, 4] print(solve(nums))
Input
[1, 0, 3, 2, 5, 4]
Output
True
Advertisements