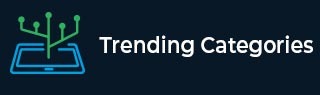
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if it is possible to serve customer queue with different notes in Python
Suppose we have an array called notes represents different rupee notes holding by customers in a queue. They are all waiting to buy tickets worth Rs 50. Here possible notes are [50, 100 and 200]. We have to check whether we can sell tickets to the people in order or not, initially we have Rs 0 in our hand.
So, if the input is like notes = [50, 50, 100, 100], then the output will be True for first two we do not need to return anything, but now we have two Rs 50 notes. So for last two we can return them Rs 50 notes and sell all tickets in order.
To solve this, we will follow these steps −
- freq := an empty map
- i := 0
- while i < size of notes, do
- if notes[i] is same as 50, then
- freq[50] := freq[50] + 1
- otherwise when notes[i] is same as 100, then
- freq[100] := freq[100] + 1
- if freq[50] is 0, then
- come out from loop
- freq[50] := freq[50] - 1
- otherwise,
- if freq[100] > 0 and freq[50] > 0, then
- freq[100] := freq[100] - 1
- freq[50] := freq[50] - 1
- otherwise when freq[50] >= 3, then
- freq[50] := freq[50] - 3
- otherwise,
- come out from loop
- if freq[100] > 0 and freq[50] > 0, then
- i := i + 1
- if notes[i] is same as 50, then
- if i is same as size of notes , then
- return True
- return False
Example
Let us see the following implementation to get better understanding −
from collections import defaultdict def solve(notes): freq = defaultdict(int) i = 0 while i < len(notes): if notes[i] == 50: freq[50] += 1 elif notes[i] == 100: freq[100] += 1 if freq[50] == 0: break freq[50] -= 1 else: if freq[100] > 0 and freq[50] > 0: freq[100] -= 1 freq[50] -= 1 elif freq[50] >= 3: freq[50] -= 3 else: break i += 1 if i == len(notes): return True return False notes = [50, 50, 100, 100] print(solve(notes))
Input
[50, 50, 100, 100]
Output
True
Advertisements