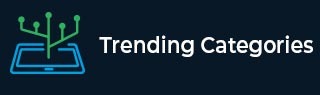
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if characters of a string can be made non-decreasing by replacing ‘_’s
In this article, we'll delve into an intriguing problem in the field of string manipulation: how to check if the characters of a given string can be made non-decreasing by replacing '?' characters. This problem provides an excellent opportunity to hone your skills in string manipulation and condition checking in C++.
Problem Statement
Given a string consisting of alphabetic characters and question marks (?), determine whether the characters can be made non-decreasing by replacing the '?'s.
The non-decreasing condition means that for every two adjacent characters in the string, the ASCII value of the second character is not less than the ASCII value of the first one.
Approach
We'll use a simple approach to solve this problem −
Iterate through the string from left to right.
If a '?' is encountered, replace it with the character that came before it (unless it's the first character, in which case replace it with 'a').
Finally, check if the resultant string is non-decreasing.
Example
We'll use a simple approach to solve this problem −
#include <stdio.h> #include <stdbool.h> #include <string.h> // Function to check if a string can be made non-decreasing by replacing '?'s bool checkNonDecreasing(char *s) { int n = strlen(s); // If the first character is '?', replace it with 'a' if (s[0] == '?') s[0] = 'a'; // Iterate through the string for (int i = 1; i < n; i++) { // If the current character is '?', replace it with the previous character if (s[i] == '?') s[i] = s[i-1]; // If the current character is less than the previous character, the string cannot be made non-decreasing if (s[i] < s[i-1]) return false; } return true; // String can be made non-decreasing } int main() { char s[] = "ac?xy"; bool result = checkNonDecreasing(s); // Print the result if (result) printf("Yes, the string can be made non-decreasing by replacing '?'s.\n"); else printf("No, the string cannot be made non-decreasing by replacing '?'s.\n"); return 0; }
Output
Yes, the string can be made non-decreasing by replacing '?'s.
#include<bits/stdc++.h> using namespace std; // Function to check if a string can be made non-decreasing by replacing '?'s bool checkNonDecreasing(string s) { int n = s.size(); // If the first character is '?', replace it with 'a' if (s[0] == '?') s[0] = 'a'; // Iterate through the string for (int i = 1; i < n; i++) { // If the current character is '?', replace it with the previous character if (s[i] == '?') s[i] = s[i-1]; // If the current character is less than the previous character, the string cannot be made non-decreasing if (s[i] < s[i-1]) return false; } return true; // String can be made non-decreasing } int main() { string s = "ac?xy"; bool result = checkNonDecreasing(s); // Print the result if(result) cout << "Yes, the string can be made non-decreasing by replacing '?'s.\n"; else cout << "No, the string cannot be made non-decreasing by replacing '?'s.\n"; return 0; }
Output
Yes, the string can be made non-decreasing by replacing '?'s.
public class Main { // Function to check if a string can be made non-decreasing by replacing '?'s public static boolean checkNonDecreasing(String s) { int n = s.length(); char[] arr = s.toCharArray(); // If the first character is '?', replace it with 'a' if (arr[0] == '?') arr[0] = 'a'; // Iterate through the string for (int i = 1; i < n; i++) { // If the current character is '?', replace it with the previous character if (arr[i] == '?') arr[i] = arr[i-1]; // If the current character is less than the previous character, the string cannot be made non-decreasing if (arr[i] < arr[i-1]) return false; } return true; // String can be made non-decreasing } public static void main(String[] args) { String s = "ac?xy"; boolean result = checkNonDecreasing(s); // Print the result if (result) System.out.println("Yes, the string can be made non-decreasing by replacing '?'s."); else System.out.println("No, the string cannot be made non-decreasing by replacing '?'s."); } }
Output
Yes, the string can be made non-decreasing by replacing '?'s.
# Function to check if a string can be made non-decreasing by replacing '?'s def checkNonDecreasing(s): n = len(s) s = list(s) # If the first character is '?', replace it with 'a' if s[0] == '?': s[0] = 'a' # Iterate through the string for i in range(1, n): # If the current character is '?', replace it with the previous character if s[i] == '?': s[i] = s[i-1] # If the current character is less than the previous character, the string cannot be made non-decreasing if s[i] < s[i-1]: return False return True # String can be made non-decreasing s = "ac?xy" result = checkNonDecreasing(s) # Print the result if result: print("Yes, the string can be made non-decreasing by replacing '?'s.") else: print("No, the string cannot be made non-decreasing by replacing '?'s.")
Output
Yes, the string can be made non-decreasing by replacing '?'s.
The checkNonDecreasing function takes as input a string s and returns a boolean value indicating whether the characters of the string can be made non-decreasing by replacing '?'s.
In this test case, the input string is "ac?b". The checkNonDecreasing function is called with this string as the argument, and the result is a boolean value that is printed out.
Conclusion
Checking if characters of a string can be made non-decreasing by replacing '?'s is a problem that tests your understanding of string manipulation and ASCII values. By practicing problems like this, you can strengthen your string handling capabilities in C++.