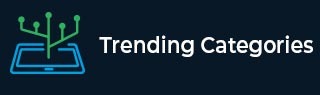
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if bitwise AND of any subset is power of two in Python
Suppose we have an array of numbers called nums. We have to check whether there exists any subset of the nums whose bitwise AND is a power of two or not.
So, if the input is like nums = [22, 25, 9], then the output will be True, as a subset {22, 9} the binary form is {10110, 1001} the AND of these two is 10000 = 16 which is power of 2.
To solve this, we will follow these steps −
- MAX := 32 considering there are 32 bits numbers at max
- Define a function solve() . This will take nums
- if size of nums is 1, then
- return true when nums[0] is power of 2, otherwise false
- total := 0
- for i in range 0 to MAX - 1, do
- total := total OR 2^i
- for i in range 0 to MAX - 1, do
- ret := total
- for j in range 0 to size of nums, do
- if nums[j] AND (2^i) is non-zero, then
- ret := ret AND nums[j]
- if nums[j] AND (2^i) is non-zero, then
- if ret is power of 2, then
- return True
- return False
Let us see the following implementation to get better understanding −
Example
MAX = 32 def is_2s_pow(v): return v and (v & (v - 1)) == 0 def solve(nums): if len(nums) == 1: return is_2s_pow(nums[0]) total = 0 for i in range(0, MAX): total = total | (1 << i) for i in range(0, MAX): ret = total for j in range(0, len(nums)): if nums[j] & (1 << i): ret = ret & nums[j] if is_2s_pow(ret): return True return False nums = [22, 25, 9] print(solve(nums))
Input
[22, 25, 9]
Output
True
Advertisements