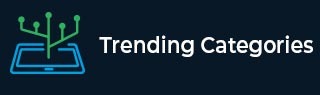
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if bits in range L to R of two numbers are complement of each other or not in Python
Suppose we have two numbers x and y and a given range (left, right). We have to check whether all bits in range left to right in both the given numbers are complement of each other or not. We have to keep in mind that from right to left, so the least significant bit is considered to be at first position.
So, if the input is like x = 41 y = 54 left = 2 right = 5, then the output will be True, as binary representation of 41 and 54 are 101001 and 110110. The bits in range 2 to 5 of x and y are "1001" and "0110" which are complement of each other.
To solve this, we will follow these steps −
- temp := x XOR y
- return true when all bits in range (left, right) of temp is 1, otherwise false
Let us see the following implementation to get better understanding −
Example
def are_all_setbits_in_range(n, left, right): val = ((1 << right) - 1) ^ ((1 << (left - 1)) - 1) new_value = n & val if val == new_value: return True return False def solve(x, y, left, right): temp = x ^ y return are_all_setbits_in_range(temp, left, right) x = 41 y = 54 left = 2 right = 5 print(solve(x, y, left, right))
Input
41, 54, 2, 5
Output
True
Advertisements