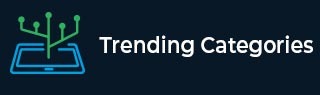
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if all the 1s in a binary string are equidistant or not in Python
Suppose we have a binary string str, we have to check whether all of the 1s in the string are equidistant or not. In other words, the distance between every two 1s is same. And the string contains at least two 1s.
So, if the input is like s = "100001000010000", then the output will be True as the 1s are at distance 4 from each other.
To solve this, we will follow these steps −
- index := a new list
- for i in range 0 to size of s, do
- if s[i] is same as 1, then
- insert i at the end of index
- if s[i] is same as 1, then
- t := size of index
- for i in range 1 to t - 1, do
- if (index[i] - index[i - 1]) is not same as (index[1] - index[0]), then
- return False
- if (index[i] - index[i - 1]) is not same as (index[1] - index[0]), then
- return True
Let us see the following implementation to get better understanding −
Example
def solve(s): index = [] for i in range(len(s)): if s[i] == '1': index.append(i) t = len(index) for i in range(1, t): if (index[i] - index[i - 1]) != (index[1] - index[0]): return False return True s = "100001000010000" print(solve(s))
Input
"100001000010000"
Output
True
Advertisements