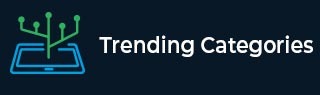
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if a string can be obtained by rotating another string 2 places in Python
Suppose we have two strings s and t. We have to check whether we can get s by rotating t two place at any direction left or right.
So, if the input is like s = "kolkata" t = "takolka", then the output will be True as we can rotate "takolka" to the left side two times to get "kolkata".
To solve this, we will follow these steps −
- if size of s is not same as size of t, then
- return False
- right_rot := blank string
- left_rot := blank string
- l := size of t
- left_rot := left_rot concatenate t[from index l - 2 to end] concatenate t[from index 0 to l - 3]
- right_rot := right_rot concatenate t[from index 2 to end] concatenate t[from index 0 to 1]
- return true when (s is same as right_rot or s is same as left_rot) otherwise false
Let us see the following implementation to get better understanding −
Example
def solve(s, t): if (len(s) != len(t)): return False right_rot = "" left_rot = "" l = len(t) left_rot = (left_rot + t[l - 2:] + t[0: l - 2]) right_rot = right_rot + t[2:] + t[0:2] return (s == right_rot or s == left_rot) s = "kolkata" t = "takolka" print(solve(s, t))
Input
"kolkata", "takolka"
Output
True
Advertisements