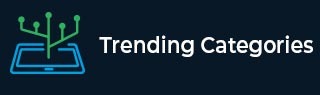
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if a number has bits in alternate pattern - Set 1 in C++
Let us consider we have an integer n. The problem is to check, whether this integer has alternate patterns in its binary equivalent or not. The alternate pattern means 101010….
The approach is like: check each digit using binary equivalent, and if two consecutive are same, return false, otherwise true.
Example
#include <iostream> using namespace std; bool hasAlternatePattern(unsigned int n) { int previous = n % 2; n = n/2; while (n > 0) { int current = n % 2; if (current == previous) // If current bit is same as previous return false; previous = current; n = n / 2; } return true; } int main() { unsigned int number = 42; if(hasAlternatePattern(number)) cout << "Has alternating pattern"; else cout << "Has no alternating pattern"; }
Output
Has alternating pattern
Advertisements