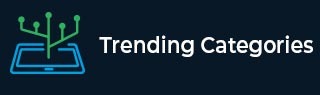
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if a line at 45 degree can divide the plane into two equal weight parts in C++
Suppose we have n different points (Xi, Yi) in 2D coordinate and each point has a weight Wi, we have to check whether a line at 45 degree can be drawn. So that the sum of weights of points on each side will be same.
So, if the input is like[[-1,1,3],[-2,1,1],[1,-1,4]], then the output will be True/
To solve this, we will follow these steps −
- n := size of v
- Define one map weight_at_x
- max_x := -2000, min_x := 2000
- for initialize i := 0, when i < n, update (increase i by 1), do −
- temp_x := v[0, i] - v[1, i]
- max_x := maximum of max_x and temp_x
- min_x := minimum of min_x and temp_x
- weight_at_x[temp_x] := weight_at_x[temp_x] + v[2, i]
- Define an array sum_temp
- insert 0 at the end of sum_temp
- for initialize x := min_x, when x <= max_x, update (increase x by 1), do −
- insert (last element of sum_temp + weight_at_x[x]) at the end of sum_temp
- total_sum := last element of sum_temp
- partition_possible := false
- for initialize i := 1, when i < size of sum_temp, update (increase i by 1), do −
- if sum_temp[i] is same as total_sum - sum_temp[i], then −
- partition_possible := true
- if sum_temp[i - 1] is same as total_sum - sum_temp[i], then −
- partition_possible := true
- if sum_temp[i] is same as total_sum - sum_temp[i], then −
- return partition_possible
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; void is_valid_part(vector<vector<int>> &v){ int n = v.size(); map<int, int> weight_at_x; int max_x = -2000, min_x = 2000; for (int i = 0; i < n; i++) { int temp_x = v[0][i] - v[1][i]; max_x = max(max_x, temp_x); min_x = min(min_x, temp_x); weight_at_x[temp_x] += v[2][i]; } vector<int> sum_temp; sum_temp.push_back(0); for (int x = min_x; x <= max_x; x++) { sum_temp.push_back(sum_temp.back() + weight_at_x[x]); } int total_sum = sum_temp.back(); int partition_possible = false; for (int i = 1; i < sum_temp.size(); i++) { if (sum_temp[i] == total_sum - sum_temp[i]) partition_possible = true; if (sum_temp[i - 1] == total_sum - sum_temp[i]) partition_possible = true; } printf(partition_possible ? "TRUE" : "FALSE"); } int main() { vector<vector<int>> v = {{-1,1,3},{-2,1,1},{1,-1,4}}; is_valid_part(v); }
Input
{{-1,1,3},{-2,1,1},{1,-1,4}}
Output
TRUE
Advertisements