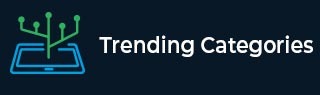
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check if a binary string contains consecutive same or not in C++
Suppose we have a binary string. Our task is to check whether the string has consecutive same characters or not. If there are consecutive same characters, then that is invalid, otherwise valid. Then the string “101010” is valid, but “10111010” is invalid.
To solve this problem, we will traverse from left to right, if two consecutive characters are the same, then return false, otherwise true.
Example
#include <iostream> #include <algorithm> using namespace std; bool isConsecutiveSame(string str){ int len = str.length(); for(int i = 0; i<len - 1; i++){ if(str[i] == str[i + 1]) return false; } return true; } int main() { string str = "101010"; if(isConsecutiveSame(str)) cout << "No consecutive same characters"; else cout << "Consecutive same characters found"; }
Output
No consecutive same characters
Advertisements