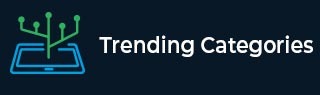
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check for the existence of key in an object using AngularJS
Overview
The existence of a key in an object can be checked using AngularJS. The key in an object is unique and cannot be duplicated. As object is a collection of different data types in Key-Value form, which contains String, Number type of data into a single reference variable. To check the existence of a key, we will use the "in" operator, which will check the object and return true or false.
Syntax
The syntax used in this problem is −
if (key in objectName ) { action } else { action }
objectName − This is the name of the object that contains the key-value pair.
key − It is the key which is searched in the object.
in − It is the “in” operator that iterates upon the given object name to check for the given key.
Explanation
In this approach, we have used the "in" operator to check for its existence. As AngularJs makes our work easy by using the expression in between HTML by using "{{ expression }}", In the script tag we had create angular module and created a object as we create normally. Then we store the input from the input field in the ng-model in the variable "val." Then the "val" is checked through the "in" operator; if the key is found in the object, it returns the if action; otherwise, another action is performed.
<script> var app = angular.module("myapp", []); app.controller("controller", function ($scope) { $scope.obj = { 'obj1': 1, 'obj2': 2, 'obj3': 3 }; $scope.checkKey = function () { var val = $scope.objKey; if (val in $scope.obj) { $scope.output = "key "+val+" exist" } else { $scope.output = "key do not exist"; } } }) </script>
Algorithm
Step 1 − Create an angular.module container, pass the name of the root element of HTML and store it in a variable.
Step 2 − Pass the $scope in the controller. Create an object with the name obj and pass another scope binding in the controller to create a function as checkKey().
Step 3 − Store a key in a variable which you have to search.
Step 4 − Use the “in” operator to iterate over the object to find the key.
Step 5 − If on iterating over objects the "in" operator finds the key which is equal to the key which we are searching for then it returns true, otherwise it returns false.
Example
<!DOCTYPE html> <html lang="en"> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.13/angular.min.js"></script> <title>How to check the existence of key in an object using AngularJS</title> <style> body{ display: flex; min-height: 90vh; place-content: center; align-items: center; background-color: #0a0a0a; color: white; } .main{ box-shadow: 0 0 8px white; padding: 0.2rem; border-radius: 5px; margin: 0 auto; } </style> </head> <body> <div ng-app="myapp"> <div ng-controller="controller" class="main"> <p>object: {{obj}}</p> <input type="text" ng-model="objKey" placeholder="Enter key here"> <button ng-click="checkKey()" style="margin-top: 5px;">Check Now</button> <p>{{output}}</p> </div> </div> <script> var app = angular.module("myapp", []); app.controller("controller", function ($scope) { $scope.obj = { 'obj1': 1, 'obj2': 2, 'obj3': 3 }; $scope.checkKey = function () { var val = $scope.objKey; if (val in $scope.obj) { $scope.output = "key "+val+" exist" } else { $scope.output = "Not Exist"; } } }) </script> </body> </html>
The output shows that we have checked for the "obj1", so this value is passed in the checkKey() function and checked for the key in the given object. It had returned true after checking through the "in" operator, and if the condition had executed with the output "key obj1 exists,"
It shows that the key that was entered in the input field was not found in the object, so it has returned false and the else condition has executed with the output "Key does not exist."
Conclusion
The return type of “in” operator is Boolean, when the particular key is found in the object it returns true otherwise false. The “in” operator can be used in JavaScript as well as in AngularJs. The object in the preceding approaches is rendered dynamically from internal javascript and inserted as an expression inside expression, but in order to use these, the parent tag must use ng-app= "" directives.