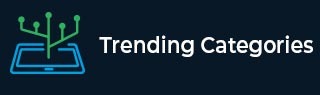
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check for Subarray in the original array with 0 sum JavaScript
We are required to write a JavaScript function that takes in an array of Numbers with some positive and negative values. We are required to determine whether there exists a subarray in the original array whose net sum is 0 or not.
Our function should return a boolean on this basis.
Approach
The approach here is simple. We iterate over the array using a for loop, calculate the cumulative sum up to that particular element. And if any point the cumulative becomes 0 or attains a value it has previously attained, then there exists a subarray with sum 0. Otherwise there exists no subarray with sum 0.
Therefore, let's write the code for this function −
Example
const arr = [4, 2, -1, 5, -2, -1, -2, -1, 4, -1, 5, -2, 3]; const zeroSum = arr => { const map = new Map(); let sum = 0; for(let i = 0; i < arr.length; i++){ sum += arr[i]; if(sum === 0 || map.get(sum)){ return true; }; map.set(sum, i); }; return false; }; console.log(zeroSum(arr));
Output
The output in the console will be −
true
Advertisements