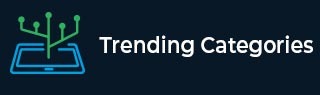
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Check for Power of two in JavaScript
We are required to write a function, say isPowerOfTwo() that takes in a positive number and returns a boolean based on the fact whether or not the number is some power of 2.
For example −
console.log(isPowerOfTwo(3)); //false console.log(isPowerOfTwo(32)); //true console.log(isPowerOfTwo(2048)); //true console.log(isPowerOfTwo(256)); //true console.log(isPowerOfTwo(22)); //false
Let’s write the code for this function, it will be a very straightforward recursive function that keeps recurring until the number stays divisible by 2, if in this process the number gets reduced all the way down to 1, it is a power of 2 otherwise it isn’t. Here is the code −
Example
const isPowerOfTwo = num => { if(num === 1){ return true; }; if(num % 2 !== 0){ return false; } return isPowerOfTwo(num / 2); } console.log(isPowerOfTwo(3)); console.log(isPowerOfTwo(32)); console.log(isPowerOfTwo(2048)); console.log(isPowerOfTwo(256)); console.log(isPowerOfTwo(22));
Output
The output in the console will be −
false true true true false
Advertisements