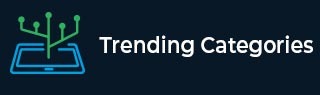
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C# Program to Generate Marksheet of Student
Introduction
There are a lot of ways to generate a mark sheet for a student. Today we will learn one of them. In this article, we will learn a C# program to generate the mark sheet of a student. Let us learn the algorithm first.
Algorithm
Let us discuss the algorithm to write the program which generates the mark sheet of a student. The student has to give the input to the system and then the mark sheet is generated.
Step 1 − Firstly we declare the integer variables for storing the different data such as the roll number of the student and marks of the subject.
Step 2 − Next we declare a float variable to store the percentage of the student.
Step 3 − Next we declare a string variable to store the name of the student.
Step 4 − Now we get the inputs from the user i.e., the student’s roll number, name, and marks in the subjects.
Step 5 − Then we add the marks of the subject and calculate the percentage of the student. We are using Convert.ToInt32() which is an explicit conversion to convert the input into a 32-bit signed integer.
Step 6 − Now, we compare the percentage with the grade chart and tell the grade of the student and close the program.
Now, it is time to see the code for the algorithm.
Example
// C# program to generate the mark sheet of the student using System; using System.Collections.Generic; using System.Linq; using System.Text; class tpt{ static void Main(string[] args) { // Variables declaration for collection of different data int rollnum, marksub1, marksub2, marksub3, marksub4, marksub5, totalmark; // Declaration of percentage variable float stud_percent; // Declaration of string variable to store student name string name; // Getting the student’s roll number Console.WriteLine("Enter the roll number of the student :"); rollnum = Convert.ToInt32(Console.ReadLine()); // Getting the student’s name Console.WriteLine("Enter the name of the student :"); name = Console.ReadLine(); // Getting the student’s first subject marks Console.WriteLine("Enter the marks of first subject : "); marksub1 = Convert.ToInt32(Console.ReadLine()); //Getting the student’s second subject marks Console.WriteLine("Enter the marks of second subject : "); marksub2 = Convert.ToInt32(Console.ReadLine()); // Getting the student’s third subject marks Console.WriteLine("Enter the marks of third subject : "); marksub3 = Convert.ToInt32(Console.ReadLine()); // Getting the student’s fourth subject marks Console.WriteLine("Enter the marks of fourth subject : "); marksub4 = Convert.ToInt32(Console.ReadLine()); // Getting the student’s fifth subject marks Console.WriteLine("Enter the marks of fifth subject : "); marksub5 = Convert.ToInt32(Console.ReadLine()); // Adding the total marks of the student totalmark = marksub1 + marksub2 + marksub3 + marksub4 + marksub5; // Calculating the percentage of the student stud_percent = totalmark / 5.0f; // Displaying the total marks and percentage of the student Console.WriteLine("Final result of {0} is : ", name); Console.WriteLine("Total Marks : " + totalmark); Console.WriteLine("Percentage : " + stud_percent); // Block to calculate the grades of the student if (stud_percent <= 35) { Console.WriteLine("Grade : F"); } else if (stud_percent >= 34 && stud_percent <= 39) { Console.WriteLine("Grade : D"); } else if (stud_percent >= 40 && stud_percent <= 59) { Console.WriteLine("Grade : C"); } else if (stud_percent >= 60 && stud_percent <= 69) { Console.WriteLine("Grade : B"); } else if (stud_percent >= 70 && stud_percent <= 79) { Console.WriteLine("Grade : B+"); } else if (stud_percent >= 80 && stud_percent <= 90) { Console.WriteLine("Grade : A"); } else if (stud_percent >= 91) { Console.WriteLine("Grade : A+"); } } }
Output
Enter the roll number of the student : 7 Enter the name of the student : Leo Enter the marks of first subject : 10 Enter the marks of second subject : 30 Enter the marks of third subject : 21 Enter the marks of fourth subject : 7 Enter the marks of fifth subject : 11 Final result of Leo is : Total Marks : 79 Percentage : 15.8 Grade : F
Now there is another way if the number of subjects is not fixed and varies along with every student then we can use the concept of arrays.
Example
// C# program to generate the mark sheet of the student using System; using System.Collections.Generic; using System.Linq; using System.Text; class tpt{ static void Main(string[] args) { // Variables declaration for collection of different data int rollnum, subnum, totalmark=0; int[] marksub=new int[25]; // Declaration of percentage variable float stud_percent; // Declaration of string variable to store student name string name; // Getting the student’s roll number Console.WriteLine("Enter the roll number of the student :"); rollnum = Convert.ToInt32(Console.ReadLine()); // Getting the student’s name Console.WriteLine("Enter the name of the student :"); name = Console.ReadLine(); // Getting the number of subjects Console.WriteLine("Enter the number of subjects : "); subnum = Convert.ToInt32(Console.ReadLine()); //Getting the student’s subject marks for(int i=1; i<=subnum; i++) { Console.WriteLine("Enter the marks of {0} subject : ",+i); marksub[i] = Convert.ToInt32(Console.ReadLine()); totalmark=totalmark+marksub[i]; } // Calculating the percentage of the student stud_percent = totalmark / subnum; // Displaying the total marks and percentage of the student Console.WriteLine("Final result of {0} is : ", name); Console.WriteLine("Total Marks : " + totalmark); Console.WriteLine("Percentage : " + stud_percent); // Block to calculate the grades of the student if (stud_percent <= 35) { Console.WriteLine("Grade : F"); } else if (stud_percent >= 34 && stud_percent <= 39) { Console.WriteLine("Grade : D"); } else if (stud_percent >= 40 && stud_percent <= 59) { Console.WriteLine("Grade : C"); } else if (stud_percent >= 60 && stud_percent <= 69) { Console.WriteLine("Grade : B"); } else if (stud_percent >= 70 && stud_percent <= 79) { Console.WriteLine("Grade : B+"); } else if (stud_percent >= 80 && stud_percent <= 90) { Console.WriteLine("Grade : A"); } else if (stud_percent >= 91) { Console.WriteLine("Grade : A+"); } } }
Output
Enter the roll number of the student : 7 Enter the name of the student : Cris Enter the number of subjects : 2 Enter the marks of 1 subject : 89 Enter the marks of 2 subject : 77 Final result of Cris is : Total Marks : 166 Percentage : 83 Grade : A
Time Complexity
The first code in which the number of subjects is known has no loops and the size of data is fixed so the time complexity is O(1).
The second code has an array for the number of subjects. So, after the number of subjects is entered the loop runs to get the marks of the subject and add them to the number of subjects entered by the user i.e., n number of times. So, the time complexity is O(N). Now, let us conclude the article.
Conclusion
In this article we learned to write a C# Program to generate a mark sheet of a student in two different ways. One with a known number of subjects and another with an unknown number of subjects. So with this, we end the article. We hope that this article enhances your knowledge regarding C#.