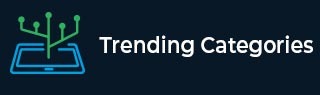
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C# Program to check if a path is a directory or a file
Introduction
Let us learn how to write C# program to check if a path is a directory or a file. A directory, also known as a folder, is a place on your computer where you can save files. A directory holds other directories or shortcuts in addition to files.
A file is a collection of data on a drive that has a unique identifier and a directory path. When a file is opened for viewing or writing, it is transformed into a stream. The stream is simply a sequence of bytes traversing the communication route.
Files vs Directory
Files are real data files, whereas directories are repositories for logical file placement on your system. To work with files and directories, the common language runtime (CLR) has the classes File, FileInfo, Directory, and DirectoryInfo in the System.IO namespace.
To deal with directories in C#, we can use Directory or DirectoryInfo. Directory class is a static class with static functions for dealing with directories. This class can not be inherited. A DirectoryInfo instance gives information about a specific directory.
There is a file class and a fileinfo class for folders. The File class is used for common tasks such as duplicating, moving, renaming, making, opening, deleting, and adding to a single file. The File class can also be used to get and change file characteristics or DateTime information associated with file creation, access, and writing. Both the File and FileInfo classes have the same fundamental functionality.
The only difference is that the File class has intrinsic methods for dealing with files, whereas the FileInfo class has instance methods for dealing with files.
Public static bool Exists (string? path); is used to check if the file or directory exists. Here the parameter is string? path. Which is the path to check. Its return type is boolean. The reason for this function to be boolean is that when the path is checked then there are only two outcomes. Either the file or directory exists or not, much like the keyword of the function. So here true is returned if there is the existence of the directory or the file and false is returned if it does not exist or any type of error occurs while trying to access the address such as a broken address or more.
Algorithm
The algorithm below will give a step-by-step procedure to write a program to check if the path given is a directory or a file.
Step 1 − Firstly we have to declare a string that contains the address which we want to check whether it is a file or directory
Step 2 − Then there has to be a condition check in which we use the method public static bool Exists (string? path); to check the existence of a file.
Step 3 − It is solely on the programmer to decide what he wants to check first. Whether he wants to check the path as a file or a directory.
Step 4 − If the path fails both of the checks then the output comes as an invalid path and the same is displayed as a message.
Example
// For File.Exists, Directory.Exists using System; using System.IO; class ttpt { static void Main() { string PathCheck = “D:/ipl”; // Here we check that the input path is a file if(File.Exists(PathCheck)) { // If the path provided is a file Console.WriteLine(“A file exists on this path”); } // Here we check if the path as input is a directory else if(Directory.Exists(PathCheck)) { // This input path is a directory Console.WriteLine(“A directory exists on this path”); } // If it is invalid i.e., it is neither directory nor a path else { Console.WriteLine("{0} is invalid. The input is neither a file nor a directory.", path); } } }
Output
A file exists on this path
The above-mentioned code is to check whether the path provided is a file or not. In the code first, we have declared a string to store the address to check whether it is a file or a directory. Then we use the public static bool Exists (string? path); which can be used with File as well as Directory class to check the existence of a file or directory by using the respective class keyword. This can be done by using the conditional check. If the person wants to do a bulk check then an array of addresses can be passed as a parameter by making a class. And then check them one by one. As public static bool Exists (string? path); returns a boolean is the reason we are doing the condition check.
Before verifying whether the directory exists, trailing spaces are deleted from the conclusion of the path argument.
The path parameter's case sensitivity correlates to the file system on which the code is executing. For example, NTFS (the usual Windows file system) is case-insensitive, whereas Linux file systems are case-sensitive.
Time Complexity
In the algorithm after the string is declared. The public static bool Exists (string? path); the method is a boolean returning method. Because it makes a single call straight to the element we are searching for, the algorithm has a time complexity of O(1).
Conclusion
So, we have reached the end of the article and we have learned how to check whether the path provided is the directory or a file. We started with the definition of the file and directory and then we moved on and saw the difference between files and directory. Then we understood the algorithm of the program and after that, we saw the program to check the path. We hope that this article enhances your knowledge regarding C#.