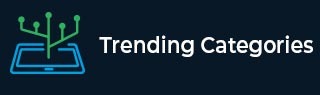
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Character class: subtraction - Java regular expressions
You can subtract one range from other and use it as new range. You can achieve this by using two variants of character classes i.e. negation and intersection.
For example the intersection of ranges [a-l] and [^e-h] gives you the characters a to l as rage subtracting the characters [e-h]
Example
import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample1 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter input text: "); String input = sc.nextLine(); String regex = "[a-l&&[^e-h]]"; //Creating a pattern object Pattern pattern = Pattern.compile(regex); //Matching the compiled pattern in the String Matcher matcher = pattern.matcher(input); int count =0; while (matcher.find()) { count++; System.out.print(matcher.group()+" "); } System.out.println("Number of matched characters: "+count); } }
Output
Enter input text: abcdefghijklmnopq a b c d i j k l Number of matched characters: 8
Advertisements