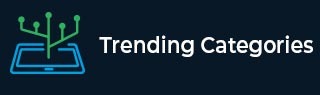
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Change a file attribute to writable in Java
The file attribute can be changed to writable using the method java.io.File.setWritable(). This method has a single parameter i.e. a boolean value that if true allows the file to be writable and if false disallows the file to be writable. Also, this method returns true if the operation is successful and false otherwise.
A program that demonstrates this is given as follows −
Example
import java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); file.setReadOnly(); System.out.println("The file can be written to? " + file.canWrite()); file.setWritable(true); System.out.println("The file can be written to? " + file.canWrite()); } catch(Exception e) { e.printStackTrace(); } } }
The output of the above program is as follows −
Output
The file can be written to? false The file can be written to? true
Note − The output may vary on Online Compilers.
Now let us understand the above program.
The attribute of a file is changed to read-only first by using the method java.io.File.setReadOnly(). Then the file attribute is changed to writable using the method java.io.File.setWritable(). The method java.io.File.canWrite() is used to check whether the file is writable or not. A code snippet that demonstrates this is given as follows −
try { File file = new File("demo1.txt"); file.createNewFile(); file.setReadOnly(); System.out.println("The file can be written to? " + file.canWrite()); file.setWritable(true); System.out.println("The file can be written to? " + file.canWrite()); } catch(Exception e) { e.printStackTrace(); }