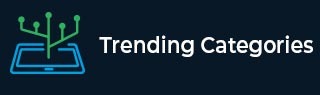
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Calculating and adding the parity bit to a binary using JavaScript
Parity Bit
A parity bit, or check bit, is a bit added to a string of bits to ensure that the total number of 1-bits in the string is even or odd.
Problem
We are required to write a JavaScript function that takes in two parameters, one being the wanted parity (always 'even' or 'odd'), and the other being the binary representation of the number we want to check.
The task of our function is to return an integer (0 or 1), which is the parity bit we need to add to the binary representation so that the parity of the resulting string is as expected.
Example
Following is the code −
const parity = 'even'; const bin = '0101010'; const findParity = (parity, bin) => { const arr = bin .toString() .split(""); let countOnes = 0; let res = 0; for (let i = 0; i < arr.length; i++) { if (arr[i] == 1) { countOnes += 1; } }; if (parity == 'even') { if (countOnes%2 == 0) { res = 0; } else { res = 1; } } else { if (countOnes%2 !== 0) { res = 0; } else { res = 1; } }; return res; }; console.log(findParity(parity, bin));
Output
1
Advertisements