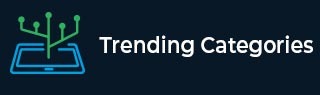
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Caesar Cipher in Cryptography
The Caesar Cipher is a simple substitution cipher named after Julius Caesar, who reportedly used it to communicate with his officials. The technique involves shifting each letter in a message by a fixed number of positions in the alphabet. For example, with a shift of 3, A would be replaced by D, B would become E, and so on.
The Caesar Cipher is relatively easy to break and is considered to be a very weak form of encryption, but it served its purpose for Julius Caesar. It's still used for educational and recreational purposes.
Algorithm for Caesar Cipher
Here is a basic algorithm for encoding a message using the Caesar Cipher with a shift of k −
Initialize a variable shift to the value of k.
Iterate through each character c in the message −
If c is a letter (uppercase or lowercase), shift it by shift positions in the alphabet.
To shift an uppercase letter, subtract 'A' from the letter, add the shift value, and take the modulus 26. Then add 'A' back to get the shifted letter.
To shift a lowercase letter, subtract 'a' from the letter, add the shift value, and take the modulus 26. Then add 'a' back to get the shifted letter.
b. Append the shifted letter to the encoded message.
Return the encoded message.
To decode an encoded message, the same algorithm can be used with a shift of -k.
Example
def caesar_cipher_encrypt(plaintext, shift): ciphertext = "" for c in plaintext: if c.isalpha(): ascii_code = ord(c) if c.isupper(): ascii_code = (ascii_code - ord('A') + shift) % 26 + ord('A') else: ascii_code = (ascii_code - ord('a') + shift) % 26 + ord('a') ciphertext += chr(ascii_code) else: ciphertext += c return ciphertext def caesar_cipher_decrypt(ciphertext, shift): plaintext = "" for c in ciphertext: if c.isalpha(): ascii_code = ord(c) if c.isupper(): ascii_code = (ascii_code - ord('A') - shift) % 26 + ord('A') else: ascii_code = (ascii_code - ord('a') - shift) % 26 + ord('a') plaintext += chr(ascii_code) else: plaintext += c return plaintext
Please note that this algorithm is limited and can be broken by a cryptanalyst with relative ease. it is not recommended to use it in any real-world applications, it's commonly used as a learning tool in the field of cryptography.
Example
Here is an example of encoding and decoding a message using the Caesar Cipher with a shift of 3 −
plaintext = "HELLO WORLD" shift = 3 ciphertext = caesar_cipher_encrypt(plaintext, shift) print("Encrypted message:", ciphertext) # Encrypted message: KHOOR ZRUOG decrypted_text = caesar_cipher_decrypt(ciphertext, shift) print("Decrypted message:", decrypted_text) # Decrypted message: HELLO WORLD
As you can see, in the first step of the process, the plaintext "HELLO WORLD" is passed to the caesar_cipher_encrypt function along with the shift value of 3, resulting in the ciphertext "KHOOR ZRUOG".
In the second step, the previously obtained ciphertext is passed to the caesar_cipher_decrypt function along with the same shift value, and the original plaintext message is obtained.
Example
plaintext = "hello world" shift = 2 ciphertext = caesar_cipher_encrypt(plaintext, shift) print("Encrypted message:", ciphertext) # Encrypted message: jgnnq ytqng decrypted_text = caesar_cipher_decrypt(ciphertext, shift) print("Decrypted message:", decrypted_text) # Decrypted message: hello world
As you can see, shift of 2 is used for the encryption and the decryption process, resulting in the same plaintext message "hello world"
Please note that this is just an example, Caesar Cipher is not secure and should not be used in real-world applications.
How to decrypt?
The Caesar Cipher is a simple substitution cipher, so the most straightforward way to decrypt an encoded message is to try different shift values until the decrypted message makes sense. This is known as a "brute-force" attack.
Here is the basic algorithm for decoding an encoded message using a brute-force attack −
Iterate through all possible shift values, starting from 0 to 25 (since there are 26 letters in the alphabet).
For each shift value, create a new empty string to hold the decoded message.
Iterate through each character c in the encoded message −
If c is a letter (uppercase or lowercase), shift it back by the current shift value positions in the alphabet
To shift an uppercase letter back, subtract the current shift value from the letter, add 26 and take modulus of 26. Then add 'A' back to get the original letter.
To shift a lowercase letter back, subtract the current shift value from the letter, add 26 and take modulus of 26. Then add 'a' back to get the original letter.
Append the shifted letter to the decoded message.
Print the decoded message along with the shift value used.
Repeat the process until the message makes sense
It's worth mentioning, that more advanced methods such as frequency analysis, pattern recognition can be used to break the ciphertext much faster than the brute force method.
It is important to note that the Caesar Cipher is very weak, and is not considered secure for use in modern cryptography. It's commonly used as a learning tool to introduce the concept of substitution ciphers.