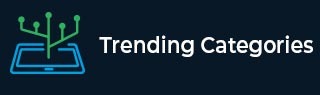
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C Program to sum the digits of a given number in single statement
In this section we will see how to find the sum of digits without writing multiple statements. In other words, we will find the sum of digits in a single statement.
As we know that, to find the sum of digits we cut the last digit by taking the remainder after dividing the number by 10, and then divide the number by 10 again and again until the number becomes 0.
To do these task in a single statement the for loop can be used. As we know there are three different sections in the for loop. In the initialization phase we are doing nothing in this case, then in the condition checking phase are checking whether the number is greater than 0 or not. In the increment decrement phase, we are doing multiple tasks. At first we are incrementing the sum by taking the last digits of the number, and also reduce the number by dividing it by 10.
Example Code
#include<stdio.h> main() { int n, sum = 0; printf("Enter a number: "); //take the number from the user scanf("%d", &n); for(; n > 0; sum += n%10, n/= 10) { } printf("The sum of digits: %d", sum); }
Output 1
Enter a number: 457 The sum of digits: 16