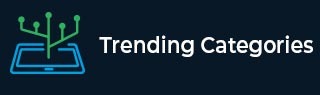
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C program to find sum, max and min with Variadic functions
Suppose we want to make some functions that can take multiple arguments, there are no fixed number of arguments. We want to make three functions sum(), max() and min(), they can calculate sum of the numbers, maximum of numbers and minimum of given numbers respectively. Each of these functions will take number of arguments count as their first argument. To define this type of functions we need to use ellipsis (...) three dots into the function argument. To use it we shall have to include stdarg.h header file. This type of function is called variadict functions. To access variable arguments there are four different things that we may notice −
va_list : This stores all given arguments
va_start : This will start accessing variable argument ap variable
va_arg : This is used to retrieve next argument of given type
va_end : This ends accessing the variable argument list
So, if we call functions like −;
- sum(5, 5, 2, 8, 9, 3)
- max(3, 5, 9, 2)
- min(6, 8, 5, 2, 6, 7, 9)
then the output will be 27 (sum of all five numbers), 9 (maximum of given three numbers), 2 (minimum of given six numbers).
To solve this, we will follow these steps −
Define a function sum (), this will take cnt, and variable number of arguments
- define va_list ap
- initialize ap by va_start(ap, cnt)
- n := 0
- for initialize i := 0, when i < cnt, update (increase i by 1), do:
- n := n + next argument by va_arg(ap, int)
- end accessing ap by va_end(ap)
- return n
- Define a function min(), this will take cnt, and variable number of arguments
- define va_list ap
- initialize ap by va_start(ap, cnt)
- minimum := 99999
- for initialize i := 0, when i < cnt, update (increase i by 1), do:
- current := next argument by va_arg(ap, int)
- if current < minimum, then:
- minimum := current
- end accessing ap by va_end(ap)
- return minimum
- Define a function max(), this will take cnt, and variable number of arguments
- define va_list ap
- initialize ap by va_start(ap, cnt)
- maximum := 0
- for initialize i := 0, when i < cnt, update (increase i by 1), do:
- current := next argument by va_arg(ap, int)
- if current > maximum, then:
- maximum := current
- end accessing ap by va_end(ap)
- return maximum
Example
Let us see the following implementation to get better understanding −
#include <stdio.h> #include <stdarg.h> int sum (int cnt,...) { va_list ap; int i, n; va_start(ap, cnt); n = 0; for (i=0;i<cnt;i++){ n += va_arg(ap, int); } va_end(ap); return n; } int min(int cnt,...) { va_list ap; int i, current, minimum; va_start(ap, cnt); minimum = 99999; for (i=0;i<cnt;i++){ current = va_arg(ap, int); if (current < minimum) minimum = current; } va_end(ap); return minimum; } int max(int cnt,...) { va_list ap; int i, current, maximum; va_start(ap, cnt); maximum = 0; for (i=0;i<cnt;i++){ current = va_arg(ap, int); if (current > maximum) maximum = current; } va_end(ap); return maximum; } int main(){ printf("%d
",sum(5, 5, 2, 8, 9, 3)); printf("%d
",max(3, 5, 9, 2)); printf("%d
",min(6, 8, 5, 2, 6, 7, 9)); }
Input
sum(5, 5, 2, 8, 9, 3) max(3, 5, 9, 2) min(6, 8, 5, 2, 6, 7, 9)
Output
27 9 2