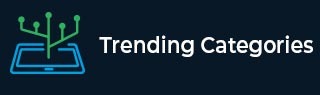
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C Program for Selection Sort?
The selection sort is assaulting algorithm that works bye buy a finding the smallest number from the array and then placing it to the first position. the next array that is to be traversed will start from index next to the position where the smallest number is placed.
Let's take an example to make this concept more clear.
We have an array {6, 3, 8, 12, 9} in this array the smallest element is 3. So we will place 3 at the first position, after this the array will look like {3, 6, 8, 12, 9}. Now we will again find the smallest number but this time we will not consider 3 in our search because it is in its place. Finding the next smallest element that is 6, creating an array with 6 at the second position and then again searching in the array till the array is sorted.
Working of selection Sort algorithm−
the following steps are followed by the selection Sort algorithm
Let's take an array {20, 12 , 23, 55 ,21}
Set the first element of the array as minimum.
Minimum = 20
Compare the minimum with the next element, if it is smaller than minimum assign this element as minimum. Do this till the end of the array.
Comparing with 12 : 20 > 12 , minimum = 12
Comparing with 23 : 12 < 23 , minimum = 12
Comparing with 55 : 12 < 55 , minimum = 12
Comparing with 21 : 12 < 21 , minimum = 12
Place the minimum at the first position( index 0) of the array.
Array = {12, 20 ,23, 55, 21}
for the next iteration, start sorting from the first unsorted element i.e. the element next to where the minimum is placed.
Array = {12, 20 ,23, 55, 21}
Searching starts from 20, next element where minimum is placed.
Iteration 2 :
Minimum = 20
Comparing with 23 : 20 < 23 , minimum = 20
Comparing with 55 : 20 < 55 , minimum = 20
Comparing with 21 : 20 < 21 , minimum = 20
Minimum in place no change,
Array = {12, 20 ,23, 55, 21}
Iteration 3 :
Minimum = 23.
Comparing with 55 : 23 < 55 , minimum = 23
Comparing with 21 : 23 > 21 , minimum = 21
Minimum is moved to index = 2
Array = {12, 20, 21, 55, 23}
Iteration 4 :
Minimum = 55
Comparing with 23 : 23 < 55 , minimum = 23
Minimum in moved to index 3 Array = {12, 20, 21, 23, 55}
Example
#include <stdio.h> int main() { int arr[10]={6,12,0,18,11,99,55,45,34,2}; int n=10; int i, j, position, swap; for (i = 0; i < (n - 1); i++) { position = i; for (j = i + 1; j < n; j++) { if (arr[position] > arr[j]) position = j; } if (position != i) { swap = arr[i]; arr[i] = arr[position]; arr[position] = swap; } } for (i = 0; i < n; i++) printf("%d\t", arr[i]); return 0; }
Output
0 2 6 11 12 18 34 45 55 99