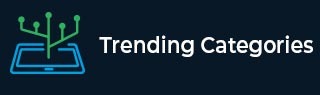
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C Program for Basic Euclidean algorithms?
Here we will see the Euclidean algorithm to find the GCD of two numbers. The GCD (Greatest Common Divisor) can easily be found using Euclidean algorithm. There are two different approach. One is iterative, another one is recursive. Here we are going to use the recursive Euclidean algorithm.
Algorithm
EuclideanAlgorithm(a, b)
begin if a is 0, then return b end if return gcd(b mod a, a) end
Example
#include<iostream> using namespace std; int euclideanAlgorithm(int a, int b) { if (a == 0) return b; return euclideanAlgorithm(b%a, a); } main() { int a, b; cout << "Enter two numbers: "; cin >> a >> b; cout << "GCD " << euclideanAlgorithm(a, b); }
Output
Enter two numbers: 12 16 GCD 4
Advertisements