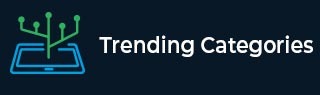
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C/C++ Program for Median of two sorted arrays of same size?
Here we will see how to get the median of two sorted array of the same size. We will use C++ STL to store array elements. After getting two arrays, we will merge them into one. As two arrays of same size are merged, then the final array will always hold even number of elements. We need to take two middle elements, then get the average of them for the median.
Algorithm
median(arr1, arr2)
Begin arr3 := array after merging arr1 and arr2 sort arr3 len := length of arr3 mid := len/2 median := (arr3[mid] + arr3[mid-1])/2 return median End
Example
#include<iostream> #include<vector> #include<algorithm> using namespace std; float median(vector<int> arr1, vector<int> arr2) { vector arr3(arr1.size() + arr2.size()); merge(arr1.begin(), arr1.end(), arr2.begin(), arr2.end(), arr3.begin()); sort(arr3.begin(), arr3.end()); int len = arr3.size(); int mid = len/2; return float(arr3[mid] + arr3[mid-1])/2; } main() { vector<int> arr1 = {1, 3, 4, 6, 7}; vector<int> arr2 = {4, 5, 7, 8, 9}; cout << "Median: " << median(arr1, arr2); }
Output
Median: 5.5
Advertisements