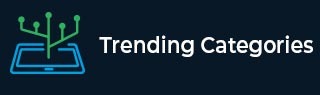
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Break a Palindrome in C++
Suppose we have a palindromic string palindrome, we have to replace exactly one character by any lowercase English letter so that the string becomes the lexicographically smallest possible string that isn't a palindrome. Now after doing so, we have to find the final string. If there is no way to do so, then return the empty string. So if the input is like “abccba”, then the output will be “aaccba”.
To solve this, we will follow these steps −
changed := false
if the size of a string is 1, then return a blank string
i := 0 and j := length of s – 1
leftA := True and rightA := True
while i < j −
if s[i] is not ‘a’, then set s[i] as ‘a’ and return s
increase i by 1 and decrease j by 1
s[size of s - 1] := ‘b’
return s
Example (C++)
Let us see the following implementation to get a better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: string breakPalindrome(string s) { bool changed = false; if(s.size() == 1)return ""; int i = 0, j = s.size() - 1; bool leftA = true; bool rightA= true; while(i < j){ if(s[i] != 'a'){ s[i] = 'a'; return s; } i++; j--; } s[s.size() - 1] = 'b'; return s; } }; main(){ Solution ob; cout << (ob.breakPalindrome("abccba")); }
Input
"abccba"
Output
aaccba