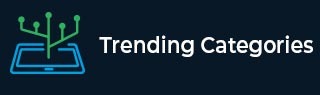
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Bisect Algorithm Functions in Python
This module provides support for maintaining a list in sorted order without having to sort the list after each insertion of new element. We will focus on two functions namely insort_left and insort_right.
insort_left
This function returns the sorted list after inserting number in the required position, if the element is already present in the list, the element is inserted at the leftmost possible position. This function takes 4 arguments, list which has to be worked with, number to insert, starting position in list to consider, ending position which has to be considered. The default value of the beginning and end position is 0 and length of the string respectively.
This is similar to inser_left except that the new element is inserted after inserting existing entries without maintaining a strict sort order.
Syntax
bisect.insort_left(a, x, lo=0, hi=len(a)) bisect.insort_left(a, x, lo=0, hi=len(a)) a is the given sequence x is the number to be inserted
Example
In the example below we see that we take a list and first apply bisect.insort_left function to it.
import bisect listA = [11,13,23,7,13,15] print("Given list:",listA) bisect.insort_left(listA,14) print("Bisect left:\n",listA) listB = [11,13,23,7,13,15] print("Given list:",listB) bisect.insort_right(listB,14,0,4) print("Bisect righ:\n",listB)
Output
Running the above code gives us the following result −
Given list: [11, 13, 23, 7, 13, 15] Bisect left: [11, 13, 23, 7, 13, 14, 15] Given list: [11, 13, 23, 7, 13, 15] Bisect righ: [11, 13, 14, 23, 7, 13, 15]