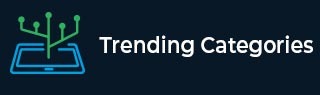
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Bind function and placeholders in C++
Here we will see the Bind function and the placeholders in C++. Sometimes we need to manipulate the operation of some functions as we need. We can use some default parameters to get some essence of manipulating.
In C++11, one new feature is introduced, called the bind function. This helps us to do such manipulating in some easier fashion. To use these features, we have to use <functional> header file.
Bind functions with the help of placeholders helps to determine the positions, and number of arguments to modify the function according to desired outputs.
Placeholders are namespaces which detect the position of a value in a function. Placeholders are represented by _1, _2, _3 etc.
Example
#include <functional> #include <iostream> struct Foo { Foo(int num) : num_(num) {} void print_add(int i) const { std::cout << num_+i << '\n'; } int num_; }; void print_num(int i) { std::cout << i << '\n'; } struct PrintNum { void operator()(int i) const { std::cout << i << '\n'; } }; int main() { std::function<void(int)> f_display = print_num; f_display(-9); std::function<void()> f_display_42 = []() { print_num(42); }; f_display_42(); std::function<void()> f_display_31337 = std::bind(print_num, 31337); f_display_31337(); std::function<void(const Foo&, int)> f_add_display = &Foo::print_add; const Foo foo(314159); f_add_display(foo, 1); std::function<int(Foo const&)> f_num = &Foo::num_; std::cout << "num_: " << f_num(foo) << '\n'; using std::placeholders::_1; std::function<void(int)> f_add_display2= std::bind( &Foo::print_add, foo, _1 ); f_add_display2(2); std::function<void(int)> f_add_display3= std::bind( &Foo::print_add, &foo, _1 ); f_add_display3(3); std::function<void(int)> f_display_obj = PrintNum(); f_display_obj(18); }
Output
-9 42 31337 314160 num_: 314159 314161 314162 18
Advertisements