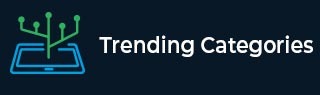
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Binary String With Substrings Representing 1 To N in C++
Suppose we have a binary string S and a positive integer N, we have to say true if and only if for every integer X from 1 to N, the binary representation of X is a substring of the given S. So if S = “0110” and N = 3, then the result will be true, as 1, 10 and 11 all are present in 0110.
To solve this, we will follow these steps −
Define a method to convert(), that will take n as input
ret := an empty string
while n is not 0
ret := ret concatenate n mod 2
n := n / 2
reverse ret and return
From the main method, do the following
for i := N, when i >= N/2, decrease i by 1
temp := convert(i)
if temp is not in S, return false
return true.
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: string convert(int n){ string ret = ""; while(n){ ret += (n % 2) + '0'; n /= 2; } reverse(ret.begin(), ret.end()); return ret; } bool queryString(string S, int N) { for(int i = N; i >= N/2; i-- ){ string temp = convert(i); if(S.find(temp) == string::npos) return false; } return true; } }; main(){ Solution ob; cout << (ob.queryString("0110", 3)); }
Input
"0110" 3
Output
1
Advertisements