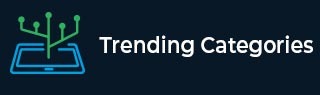
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Binary Gap in Python
Suppose we have a positive integer N, we have to find the longest distance between two consecutive 1's in the binary representation of N. If there are no two-consecutive 1's, then return 0.
So, if the input is like 22, then the output will be 2, as 22 in binary is 10110. There are three ones In the binary representation of 22, and two consecutive pairs of 1's. The first consecutive pair of 1's have distance 2, then the second consecutive pair of 1's have distance 1. Answer will be the largest of these two distances, which is 2.
To solve this, we will follow these steps −
- K := make a list of bits of binary representation of N
- Max := 0, C := 0, S := 0
- Flag := False
- for i in range 0 to size of K, do
- if K[i] is '1' and C is 0 and Flag is False, then
- C:= i
- Flag := True
- otherwise when K[i] is '1' and Flag, then
- S:= i
- if Max<abs(S-C), then
- Max := |S-C|
- C:= S
- if K[i] is '1' and C is 0 and Flag is False, then
- return Max
Let us see the following implementation to get better understanding −
Example
class Solution: def binaryGap(self, N): B = bin(N).replace('0b','') K = str(B) K = list(K) Max = 0 C = 0 S =0 Flag =False for i in range(len(K)): if K[i] is '1' and C is 0 and Flag is False: C=i Flag = True elif K[i] is '1' and Flag: S=i if Max<abs(S-C): Max = abs(S-C) C=S return Max ob = Solution() print(ob.binaryGap(22))
Input
22
Output
2
Advertisements