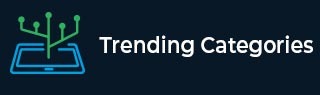
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Binary element list grouping in Python
Suppose we have a list of lists in which each sublist has two elements. One element of each sublist is common across many other subjects of the list. We need to create a final list which will show sublists grouped by common elements.
With set and map
In the given list the first element is a string and the second element is a number. So we create a temp list which will hold the second element of each sublist. Then we compare is sublist with each of the element in the temp list and designer follow to group them.
Example
listA = [['Mon', 2], ['Tue', 3], ['Wed', 3], ["Thu", 1], ['Fri', 2], ['Sat', 3], ['Sun', 1]] # With set and map temp = set(map(lambda i: i[1], listA)) res = [[j[0] for j in listA if j[1] == i] for i in temp] # Result print("The list with grouped elements is : \n" ,res)
Output
Running the above code gives us the following result −
The list with grouped elements is : [['Thu', 'Sun'], ['Mon', 'Fri'], ['Tue', 'Wed', 'Sat']]
With groupby and itemgetter
The itemgetter function is used to get the second element of each sublist. Then a group by function is applied based on the result from itemgetter function.
Example
from itertools import groupby from operator import itemgetter listA = [['Mon', 2], ['Tue', 3], ['Wed', 3],["Thu", 1], ['Fri', 2], ['Sat', 3],['Sun', 1]] # With groupby listA.sort(key = itemgetter(1)) groups = groupby(listA, itemgetter(1)) res = [[i[0] for i in val] for (key, val) in groups] # Result print("The list with grouped elements is : \n" ,res)
Output
Running the above code gives us the following result −
The list with grouped elements is : [['Thu', 'Sun'], ['Mon', 'Fri'], ['Tue', 'Wed', 'Sat']]
With defaultdict
We Apply the defaultdict function to get the second elements of the sublist as the keys of the dictionary. Then in the resulting list we append the values from the first element of the sublist.
Example
import collections listA = [['Mon', 2], ['Tue', 3], ['Wed', 3],["Thu", 1], ['Fri', 2], ['Sat', 3],['Sun', 1]] # With defaultdict res = collections.defaultdict(list) for val in listA: res[val[1]].append(val[0]) # Result print("The list with grouped elements is : \n" ,res)
Output
Running the above code gives us the following result −
The list with grouped elements is : defaultdict(<class 'list'>, {2: ['Mon', 'Fri'], 3: ['Tue', 'Wed', 'Sat'], 1: ['Thu', 'Sun']})