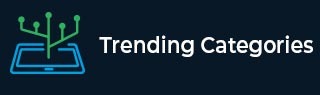
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Bash Script for Loop Explained with Examples
If you're a Linux or Unix user, chances are you've used Bash at least once or twice. Bash is a command-line shell that lets you interact with your operating system in a more direct and powerful way than using a graphical user interface. One of most powerful features of Bash is for loop, which lets you automate repetitive tasks by iterating over a list of values. In this article, we'll explore how Bash for loop works and provide some examples of how you can use it in your own scripts.
What is a For Loop?
A for loop is a control structure that lets you execute a block of code repeatedly for a fixed number of times, or over a list of values. basic syntax of a for loop is as follows −
for variable in list do commands done
Here, variable is a placeholder that takes on value of each item in list in turn, and commands is a block of Bash commands that are executed each time loop runs. loop continues until every item in list has been processed.
Iterating Over a List of Values
The simplest use case for a for loop is to iterate over a list of values. Let's say you have a list of file names in a directory, and you want to print out each name on a separate line. Here's how you would do it −
for file in /path/to/directory/* do echo $file done
In this example, we use wildcard character * to specify that we want to loop over all files in directory. echo command is used to print out each file name on a separate line. Notice that we use $ symbol to reference file variable when we call echo.
Iterating Over a Range of Numbers
Another common use case for a for loop is to iterate over a range of numbers. Let's say you want to print out numbers 1 to 10 on separate lines. Here's how you would do it −
Example
for i in {1..10} do echo $i done
In this example, we use curly braces {} to specify range of numbers we want to loop over. echo command is used to print out each number on a separate line.
Output
1 2 3 4 5 6 7 8 9 10
Iterating Over an Array
In Bash, you can also create arrays to store multiple values in a single variable. Here's an example of how you can use a for loop to iterate over an array of names −
Example
names=("Alice" "Bob" "Charlie" "Dave") for name in "${names[@]}" do echo "Hello, $name!" done
Output
Hello, Alice! Hello, Bob! Hello, Charlie! Hello, Dave!
In this example, we define an array called names that contains four values. "${names[@]}" syntax is used to expand array into a list of values that for loop can iterate over. echo command is used to print out a greeting for each name.
Nested For Loops
Sometimes you may need to use a for loop inside another for loop. This is called a nested for loop. Let's say you have a list of file extensions and a list of directories, and you want to loop over each combination of extension and directory. Here's how you would do it −
Example
extensions=("txt" "pdf" "docx") directories=("/path/to/dir1" "/path/to/dir2" "/path/to/dir3") for ext in "${extensions[@]}" do for dir in "${directories[@]}" do echo "Files with extension .$ext in $dir:" ls "$dir"/*.$ .$ext done done
In this example, we define two arrays called `extensions` and `directories`. We use a nested for loop to iterate over each combination of extension and directory. `echo` command is used to print out a message indicating extension and directory being processed, and `ls` command is used to list all files in directory with current extension.
Skipping an Iteration with Continue
Sometimes you may want to skip an iteration of for loop based on a certain condition. This can be done using `continue` keyword. Let's say you have a list of file names, and you want to print out all names except for files that start with letter "a". Here's how you would do it −
for file in /path/to/directory/* do if [[ "$file" == /path/to/directory/a* ]]; then continue fi echo $file done
In this example, we use an `if` statement to check if file name starts with letter "a". If it does, we use `continue` keyword to skip rest of code in loop and move on to next iteration. If file name does not start with "a", we use `echo` command to print out file name.
Advanced For Loop Techniques
In addition to basic syntax of for loop, Bash also provides a number of advanced techniques that you can use to make your for loops more powerful and flexible. Here are some examples −
Using Output of a Command as a List
You can use output of a command as list to iterate over in a for loop. Let's say you want to print out all directories in your home directory that have more than 10 files in them. Here's how you would do it −
for dir in $(find ~/ -type d -exec sh -c 'ls -1 "{}" | wc -l' \; -print | awk -F: '$1>=10 {print $2}') do echo $dir done
In this example, we use find command to locate all directories in our home directory. We then use a combination of sh, ls, wc, and awk commands to count number of files in each directory and filter out directories that have fewer than 10 files. resulting list of directories is then used as input to for loop.
Using a C-style For Loop
Bash also provides a way to use a C-style for loop syntax, which can be useful for more complex looping scenarios. Here's an example −
Example
for ((i=0; i<10; i++)) do echo $i done
Output
0 1 2 3 4 5 6 7 8 9
In this example, we use ((...)) syntax to define a C-style for loop that counts from 0 to 9. i++ expression is used to increment value of i by 1 each time loop runs.
Using a While Loop with a Read Command
Finally, you can use a while loop with read command to iterate over a list of values entered by user. Here's an example −
while read line do echo "You entered: $line" done
In this example, we use read command to read in a line of text entered by user. while loop then continues to run until user enters an end-of-file character (usually Ctrl+D). echo command is used to print out each line of text entered by user.
Tips For Using Bash For Loop Effectively
While Bash for loop is a powerful tool, there are some tips and tricks you can use to make your loops even more effective. Here are some things to keep in mind when using Bash for loop −
Use Descriptive Variable Names
When defining variable that holds each item in list, use a name that describes item's purpose. For example, instead of using x or i, use a name like filename or counter.
Use Quotes Around Variable References
When referencing variable that holds each item in list, always use quotes around reference to ensure that script works correctly even if item contains spaces or other special characters.
Use a Counter Variable For Complex Iterations
When iterating over multiple lists or performing complex calculations in a loop, use a separate counter variable to keep track of current iteration number. This can make loop easier to read and debug.
Avoid Infinite Loops
Always ensure that your loop has a clear termination condition to avoid an infinite loop. For example, if you are iterating over a range of numbers, ensure that loop terminates when it reaches end of range.
Use Conditional Statements When Necessary
If you need to skip certain iterations or terminate loop early based on a certain condition, use conditional statements like if and break to control flow of loop.
By following these tips, you can write more efficient and effective Bash for loops that accomplish your tasks quickly and accurately.
Conclusion
The Bash for loop is a powerful tool for automating repetitive tasks in Linux and Unix environments. By using a for loop, you can easily iterate over a list of values, a range of numbers, or an array. You can also use nested for loops to iterate over multiple lists simultaneously. Additionally, you can use `continue` keyword to skip iterations based on a certain condition. With examples provided in this article, you should be able to start using Bash for loop in your own scripts and make your work more efficient and streamlined.