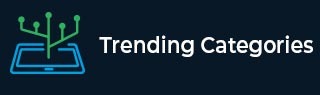
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Average of array excluding min max JavaScript
In this problem statement, our task is to find the average of the array excluding minimum and maximum values from the array with the help of Javascript functionalities. This task can be done by excluding the minimum and maximum values and calculating the average of the rest of the elements.
Logic for the given problem
To create the program for this problem we will use the Math function of Javascript to get the minimum and maximum values with the help of min and max keywords.
After we are having the values of min and max now we will filter the array and exclude these values from the array. And calculate the average for remaining values with the reduce method.
Algorithm
Step 1 − At the beginning, we will create a function to calculate the average of array elements and pass an array in it.
Step 2 − As we have ro exclude the min and max values from the array. This step will find out the minimum and maximum items of the given array. And to get these values we will use Math function and min and max keywords.
Step 3 − After having values of min and max, its time to exclude them from the array because we have to calculate the average of remaining items of the array. For doing this process we will use a filter method to filter out these elements.
Step 4 − As we have already excluded the min and max values of the array, now we will calculate the sum of the rest of the elements of the array. And for summing up the rest of the items we will use the reduce method.
Step 5 − Now we have sum of the rest of the elements, now divide it to the length of the remaining elements to get the average of them.
Step 6 − At the end, return the output as average of the rest of the elements.
Code for the algorithm
function average(arr) { const min = Math.min(...arr); const max = Math.max(...arr); //filter the input array and exclude min and max values const filterArr = arr.filter(x => x !== min && x !== max); const sum = filterArr.reduce((acc, val) => acc + val, 0); return sum / filterArr.length; } const arr = [10, 20, 30, 40, 50]; const avg = average(arr); console.log(avg);
In the above code we have used some built in functions of Javascript to make the code easy and fast. With the help of the Math function we have found the min and max values. And the filter method filters them from the array. Lastly, the reduce method is calculating the sum of the rest of the items.
So our output is 30, because the average of the remaining elements 20, 30, 40 is 30. Here 10 is the smallest element of the array and 50 is the largest element of the array. As per the problem statement we have excluded these two elements to get the required result.
Complexity
Let's say n is the length of the input array, so the time complexity of the above code is O(n). Because we have iterated over all the elements multiple times but every iteration is about linear time. And the space complexity for the above code is O(n) as we have created a new array to memorize the filtered items.
Conclusion
This is the way we can solve the above problem. We have basically used reduce, Math functions of Javascript to get the required result. The problem stated that we have to exclude the minimum and maximum values and show the average of remaining elements. So the time taken to execute the problem is O(n) as we have n items in the array.