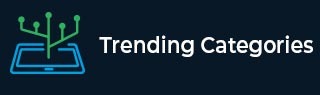
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Arnab Chakraborty has Published 4452 Articles

Arnab Chakraborty
122 Views
Suppose we have a binary search tree and a node in it, we have to search the in-order successor of that node in the BST. As we know that the successor of a node p is the node with the smallest key greater than p.val.So, if the input is like ... Read More

Arnab Chakraborty
183 Views
Suppose there are two 1D arrays, we have to implement an iterator that will return their elements alternately. There will be two methods −next() − to get next elementhasNext() − to check whether the next element is present or not.So, if the input is like v1 = [1, 2] v2 ... Read More

Arnab Chakraborty
797 Views
Suppose we have an unsorted array called nums, we have to reorder it in-place such that nums[0] = nums[2] nums[i+1] is true or i is odd and nums[i] > nums[i+1] is false, thenswap(nums[i], nums[i + 1])Example Let us see the following implementation to get better understanding − Live Demo#include using ... Read More

Arnab Chakraborty
94 Views
Suppose we have n people (labeled from 0 to n - 1) and among them, there may exist one celebrity. We can say a person x is a celebrity when all the other n - 1 people know x but x does not know any of them. Here we have ... Read More

Arnab Chakraborty
4K+ Views
Suppose we have a list of strings. We have to design an algorithm that can encode the list of strings to a string. We also have to make one decoder that will decode back to the original list of strings. Suppose we have the encoder and decoder installed on these ... Read More

Arnab Chakraborty
156 Views
Suppose we have a string s, we have to find all the palindromic permutations of it, there will be no repetition. If no palindromic permutation is there, then simply return empty string.So, if the input is like "aabb", then the output will be ["abba", "baab"]To solve this, we will follow ... Read More

Arnab Chakraborty
222 Views
Suppose we have n nodes they are labeled from 0 to n-1 and a list of undirected edges [u, v], We have to define a function to check whether these edges make up a valid tree or not.So, if the input is like n = 5, and edges = [[0, ... Read More

Arnab Chakraborty
239 Views
Suppose we have an array of n integers called nums and we also have a target, we have to find the number of index triplets (i, j, k) here i, j, k all are in range 0 to n - 1 and that satisfy the condition nums[i] + nums[j] + ... Read More

Arnab Chakraborty
95 Views
Suppose we have a sequence of numbers; we have to check whether it is the correct preorder traversal sequence of a binary search tree. We can assume that each number in the sequence is unique. Consider the following binary search tree −So, if the input is like [5, 2, 1, ... Read More

Arnab Chakraborty
197 Views
Suppose we have a number. The numbers can be regarded as a product of its factors. So, 8 = 2 x 2 x 2; = 2 x 4. We have to make one function that takes an integer n and return all possible combinations of its factors.So, if the input ... Read More