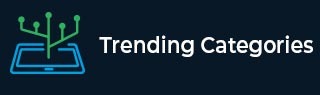
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
AmitDiwan has Published 11365 Articles

AmitDiwan
77 Views
Let’s say the following is our string −String str = "My String";Now, convert the above to IntStream −IntStream stream = str.chars();ExampleFollowing is the program to convert String to IntStream in Java −import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { String str = ... Read More

AmitDiwan
80 Views
Let’s first create an IntStream −IntStream stream = "Ryan".chars();Now, convert this IntStream to String −String str = stream.collect(StringBuilder::new, StringBuilder::appendCodePoint, StringBuilder::append).toString();ExampleFollowing is the program to convert IntStream to String in Java −import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { IntStream stream = "Ryan".chars(); ... Read More

AmitDiwan
619 Views
At first create a HashMap −Map map = new HashMap(); map.put("1", "One"); map.put("2", "Two"); map.put("3", "Three"); map.put("4", "Four"); map.put("5", "Five"); map.put("6", "Six");Now, convert the above HashMap to TreeMap −Map treeMap = new TreeMap(); treeMap.putAll(map);ExampleFollowing is the program to convert HashMap to TreeMap in Java −import java.util.*; import java.util.stream.*; public class ... Read More

AmitDiwan
108 Views
A boxed array is an array which is defined in the form of an object, instead of the primitives.ExampleFollowing is the program to convert Boxed Array to Stream in Java −import java.util.*; import java.util.stream.*; public class Demo { public static void main(String args[]) { String arr[] ... Read More

AmitDiwan
100 Views
Iterator in Java is used to traverse each and every element in the collection. Using it, traverse, obtain each element or you can even remove elements from ArrList. To use an iterator to cycle through the contents of a collection, at first obtain an iterator to the start of the ... Read More

AmitDiwan
685 Views
At first, let us set an ArrayList of string −ArrayList arrList = new ArrayList(); arrList.add("Bentley"); arrList.add("Audi"); arrList.add("Jaguar"); arrList.add("Cadillac");Now, use toArray() to convert to a string array −int size = arrList.size(); String res[] = arrList.toArray(new String[size]);ExampleFollowing is the program to convert an ArrayList of String to a String array in Java ... Read More

AmitDiwan
418 Views
At first, set a list with string values −List myList = new ArrayList( Arrays.asList("One", "Two", "Three", "Four"));Now, use String.join() to set them as a comma separated list −String str = String.join(", ", myList);ExampleFollowing is the program to convert string into comma separated List in Java −import java.util.*; public class Demo ... Read More

AmitDiwan
431 Views
At first, create a Set of string −Set setStr = new HashSet(Arrays.asList("osCommerce", "PrestaShop", "Magento", "Wordpres", "Drupal"));Now, use the toArray() method to convert to array of string −String[] arrStr = setStr.toArray(new String[0]);ExampleFollowing is the program to convert Set of String to Array of String in Java −import java.util.Arrays; import java.util.Set; import ... Read More

AmitDiwan
555 Views
Let’s say the following is our ArrayList −List arrList = Arrays.asList("John", "Jacob", "Kevin", "Katie", "Nathan");Now, convert this ArrayList to LinkedList using toCollection() −List myList = arrList.stream().collect(Collectors.toCollection(LinkedList::new));ExampleFollowing is the program to convert ArrayList to LinkedList in Java −import java.util.*; import java.util.stream.*; public class Demo { public static void main(String args[]) ... Read More

AmitDiwan
199 Views
Let’s say the following is our vector with values −Vector v = new Vector(); v.add("20"); v.add("40"); v.add("60"); v.add("80"); v.add("100");Now, convert the above Vector to List −ListmyList = new ArrayList(v);ExampleFollowing is the program to convert a Vector to List in Java −import java.util.*; public class Demo { public static void ... Read More