- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10710 Articles for Web Development

532 Views
ProblemWe are required to write a JavaScript function that takes in a string that represents a sentence. Our function should sort this sentence.Each word in the sentence string contains an integer. Our function should sort the string such that the word that contains the smallest integer is placed first and then in the increasing order.ExampleFollowing is the code − Live Democonst str = "is2 Thi1s T4est 3a"; const sortByNumber = (str = '') => { const findNumber = (s = '') => s .split('') .reduce((acc, val) => +val ? +val : acc, 0); ... Read More

80 Views
ProblemWe are required to write a JavaScript function that takes in a number. Our function should return the value of −(i)nHere,i = -11/2Therefore,i^2 = -1 i^3 = -i i^4 = 1 and so onExampleFollowing is the code − Live Democonst num = 657; const findNthPower = (num = 1) => { switch(num % 4){ case 0: return '1'; case 1: return 'i'; case 2: return '-1'; case 3: return '-i'; }; }; console.log(findNthPower(num));Outputi

980 Views
ProblemWe are required to write a JavaScript function that takes in two strings. Our function should return a new string of characters which is not common to both the strings.ExampleFollowing is the code − Live Democonst str1 = "xyab"; const str2 = "xzca"; const findUncommon = (str1 = '', str2 = '') => { const res = []; for (let i = 0; i < str1.length; i++){ if (!(str2.includes(str1[i]))){ res.push(str1[i]) } } for (let i = 0; i < str2.length; i++){ if (!(str1.includes(str2[i]))){ res.push(str2[i]) } } return res.join(""); }; console.log(findUncommon(str1, str2));Outputybzc

688 Views
ProblemWe are required to write a JavaScript function that takes in an array of strings. Our function should remove the duplicate characters that appear consecutively in the strings and return the new modified array of strings.ExampleFollowing is the code − Live Democonst arr = ["kelless", "keenness"]; const removeConsecutiveDuplicates = (arr = []) => { const map = []; const res = []; arr.map(el => { el.split('').reduce((acc, value, index, arr) => { if (arr[index] !== arr[index+1]) { map.push(arr[index]); } if (index === arr.length-1) { res.push(map.join('')); map.length = 0 } }, 0); }); return res; } console.log(removeConsecutiveDuplicates(arr));Output[ 'keles', 'kenes' ]

591 Views
ProblemWe are required to write a JavaScript function that takes in a string that might contain some spaces.Our function should reverse the words present in the string internally without interchange the characters of two separate words or the spaces.ExampleFollowing is the code − Live Democonst str = 'this is normal string'; const reverseWordsWithin = (str = '') => { let res = ""; for (let i = str.length - 1; i >= 0; i--){ if(str[i] != " "){ res += str[i]; }; if(str[res.length] == " "){ res += str[res.length]; }; }; return res; }; console.log(reverseWordsWithin(str));Outputgnir ts lamron sisiht

124 Views
ProblemWe are required to write a JavaScript function that takes in a range array of two numbers. Our function should return the count of such prime numbers whose squared sum of digits eventually yields 1.For instance, 23 is a prime number and,22 + 32 = 13 12 + 32 = 10 12 + 02 = 1Hence, 23 should be a valid number.ExampleFollowing is the code − Live Democonst range = [2, 212]; String.prototype.reduce = Array.prototype.reduce; const isPrime = (n) => { if ( n acc+v*v, 0 ))); return n===1; } const countDesiredPrimes = ([a, b]) => { let res=0; for ( ; a

120 Views
ProblemWe are required to write a JavaScript function that takes in a number.Our function should iterate through the binary equivalent of the number and swap its adjacent bits to construct a new binary. And then finally our function should return the decimal equivalent of the new binary.ExampleFollowing is the code − Live Democonst num = 13; const swapBits = (num) => { let arr = num.toString(2).split(''); if(arr.length % 2){ arr.unshift(0); } for(let i = 0; i < arr.length - 1; i = i + 2) { [arr[i], arr[i + 1]] = [arr[i + 1], arr[i]]; } return +('0b' + arr.join('')); } console.log(swapBits(num));Output14

459 Views
ProblemWe are required to write a JavaScript function that takes in a string. Our function should return the length of the longest contiguous substring that contains only vowels.ExampleFollowing is the code − Live Democonst str = 'schooeal'; const findLongestVowel = (str = '') => { let cur = 0 let max = 0 for (let i = 0; i < str.length; ++i) { if ("aeiou".includes(str[i])) { cur++ if (cur > max) { max = cur } } else { cur = 0 } } return max }; console.log(findLongestVowel(str));Output4
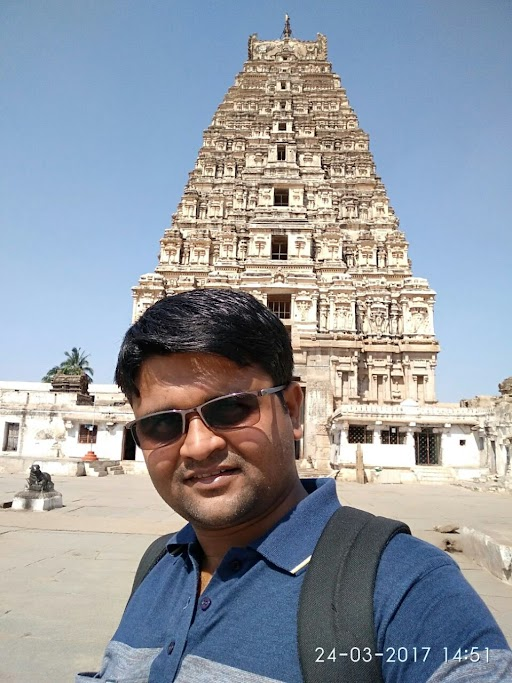
5K+ Views
Hypertext and Hyperlink are related terms associated with navigation over computer network but they have a different meaning. One major difference between the hypertext and hyperlink is that the hypertext is a data which when clicked redirects us to some other location, while the hyperlink is a link to a computer resource embedded in the hypertext. Therefore, we can state that the hyperlink is an internal part of the hypertext. In this article, we discuss all the important differences between a hypertext and a hyperlink. Let's start with some basics of hypertexts and hyperlinks. What is a Hypertext? A ... Read More

368 Views
ProblemWe are required to write a JavaScript function that takes in a number. Our function should return the corresponding ASCII alphabet for that number (if there exists an alphabet for that ASCII value), -1 otherwise.The condition here is that we cannot use any inbuilt function that converts these values.ExampleFollowing is the code − Live Democonst num = 98; const findChar = (num = 1) => { const alpha = 'abcdefghijklmnopqrstuvwxyz'; if(num >= 97 && num = 65 && num